Java
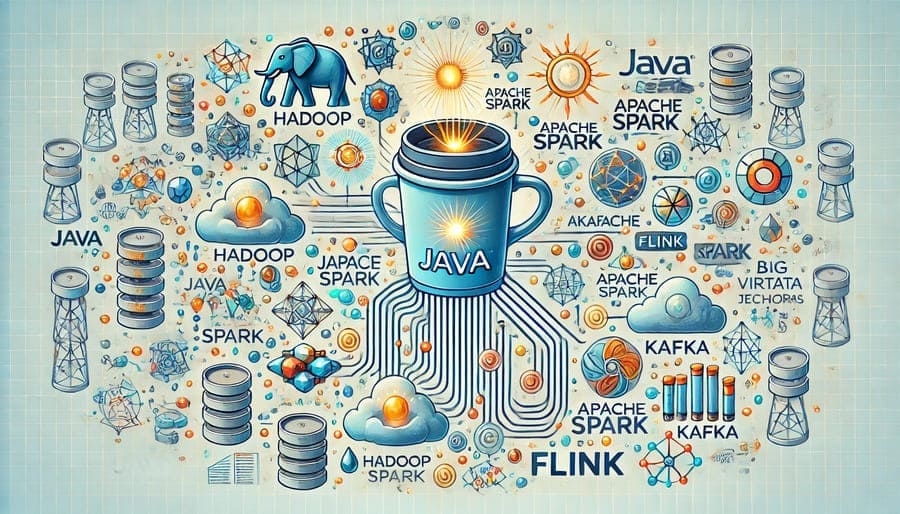
In the ever-evolving landscape of technology, certain programming languages have stood the test of time while adapting to new challenges. Java stands tall among these survivors, maintaining its position as one of the most widely used programming languages in the world. What’s particularly fascinating is how Java, created long before the concept of “big data” existed, has become the backbone of many critical big data technologies and frameworks. This comprehensive guide explores Java’s unique role in the big data ecosystem, its strengths that make it ideal for data-intensive applications, and why it continues to be the language of choice for building robust data processing systems.
When Java was introduced by Sun Microsystems in 1995, its creators couldn’t have predicted how perfectly suited it would be for big data applications that would emerge decades later. Java was designed with several core principles that would later prove invaluable for distributed data processing:
// The famous "Write Once, Run Anywhere" principle demonstrated
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello from any platform!");
}
}
Java’s platform independence through the Java Virtual Machine (JVM), robust security model, and strong focus on network programming created the perfect foundation for what was to come in the data engineering world.
The Java Virtual Machine provides a layer of abstraction between code and hardware, enabling applications to run consistently across different platforms. This “write once, run anywhere” philosophy is crucial for big data frameworks that must operate across heterogeneous clusters of machines.
// Java's ability to handle platform-specific operations through abstraction
import java.io.File;
public class FileSystemCheck {
public static void main(String[] args) {
// Works the same on Windows, Linux, macOS, etc.
File dataDirectory = new File("/data/warehouse");
long freeSpace = dataDirectory.getFreeSpace();
System.out.println("Available storage: " + (freeSpace / 1073741824) + " GB");
}
}
The JVM doesn’t just run Java code—it has become a polyglot platform supporting languages like Scala, Kotlin, and Clojure, expanding the ecosystem while maintaining compatibility.
Java’s performance characteristics make it ideal for data-intensive applications:
- Just-In-Time (JIT) compilation: Converts frequently executed bytecode to native machine code
- Advanced garbage collection: Manages memory efficiently for long-running processes
- Thread management: Provides sophisticated concurrency controls for parallel processing
// Example of Java's concurrency capabilities for parallel data processing
import java.util.Arrays;
import java.util.concurrent.ForkJoinPool;
import java.util.concurrent.RecursiveTask;
public class ParallelDataProcessor extends RecursiveTask<Long> {
private final long[] data;
private final int start;
private final int end;
private static final int THRESHOLD = 10000;
public ParallelDataProcessor(long[] data, int start, int end) {
this.data = data;
this.start = start;
this.end = end;
}
@Override
protected Long compute() {
if (end - start <= THRESHOLD) {
// Process data directly for small chunks
long sum = 0;
for (int i = start; i < end; i++) {
sum += data[i];
}
return sum;
} else {
// Split and process in parallel for large datasets
int mid = start + (end - start) / 2;
ParallelDataProcessor left = new ParallelDataProcessor(data, start, mid);
ParallelDataProcessor right = new ParallelDataProcessor(data, mid, end);
left.fork();
long rightResult = right.compute();
long leftResult = left.join();
return leftResult + rightResult;
}
}
public static void main(String[] args) {
long[] data = new long[100_000_000];
Arrays.fill(data, 1);
ForkJoinPool pool = new ForkJoinPool();
long sum = pool.invoke(new ParallelDataProcessor(data, 0, data.length));
System.out.println("Sum of 100 million elements: " + sum);
}
}
In distributed systems, failure is inevitable. Java’s exception handling system provides a structured approach to managing errors:
// Error handling in a distributed data processing context
public void processDataBatch(List<Record> batch) {
for (Record record : batch) {
try {
parseRecord(record);
transformRecord(record);
loadRecord(record);
} catch (ParseException e) {
// Handle data format errors
errorCollector.logParseError(record, e);
metrics.incrementParseErrors();
} catch (TransformException e) {
// Handle transformation errors
errorCollector.logTransformError(record, e);
metrics.incrementTransformErrors();
} catch (LoadException e) {
// Handle database errors
if (e.isRetryable()) {
retryQueue.add(record);
} else {
errorCollector.logPermanentLoadError(record, e);
}
metrics.incrementLoadErrors();
} catch (Exception e) {
// Handle unexpected errors
errorCollector.logUnexpectedError(record, e);
metrics.incrementUnexpectedErrors();
// Consider if we should stop processing this batch
if (shouldAbortBatch(metrics)) {
throw new BatchProcessingException("Too many errors, aborting batch", e);
}
}
}
}
This error resilience is essential for big data applications that must process petabytes of data without failing completely when individual records have issues.
Java’s strong typing helps catch errors at compile time rather than runtime, while its object-oriented nature encourages well-structured code:
// Strongly typed data models for reliable data processing
public class CustomerEvent {
private final String customerId;
private final EventType eventType;
private final LocalDateTime timestamp;
private final Map<String, String> properties;
// Constructor, getters, validation, etc.
public enum EventType {
PAGE_VIEW,
PURCHASE,
ACCOUNT_CREATION,
LOGIN,
LOGOUT
}
// Methods to transform, filter, or aggregate events
public boolean isConversionEvent() {
return eventType == EventType.PURCHASE;
}
public double getRevenueAmount() {
if (!isConversionEvent()) {
return 0.0;
}
String revenueStr = properties.get("amount");
return revenueStr != null ? Double.parseDouble(revenueStr) : 0.0;
}
}
These features enable developers to build complex data processing pipelines that can be maintained and evolved over time.
Java’s capabilities have made it the foundation for numerous big data technologies:
The Apache Hadoop ecosystem, which revolutionized big data processing, is primarily written in Java:
- Hadoop HDFS: Distributed file system for storing massive datasets
- Hadoop MapReduce: Framework for parallel processing of large datasets
- HBase: Distributed, scalable NoSQL database
- Hive: Data warehouse infrastructure for data summarization and querying
// Simple MapReduce job in Java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
import java.io.IOException;
import java.util.StringTokenizer;
public class WordCount {
public static class TokenizerMapper
extends Mapper<Object, Text, Text, IntWritable> {
private final static IntWritable one = new IntWritable(1);
private Text word = new Text();
public void map(Object key, Text value, Context context
) throws IOException, InterruptedException {
StringTokenizer itr = new StringTokenizer(value.toString());
while (itr.hasMoreTokens()) {
word.set(itr.nextToken());
context.write(word, one);
}
}
}
public static class IntSumReducer
extends Reducer<Text, IntWritable, Text, IntWritable> {
private IntWritable result = new IntWritable();
public void reduce(Text key, Iterable<IntWritable> values,
Context context
) throws IOException, InterruptedException {
int sum = 0;
for (IntWritable val : values) {
sum += val.get();
}
result.set(sum);
context.write(key, result);
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "word count");
job.setJarByClass(WordCount.class);
job.setMapperClass(TokenizerMapper.class);
job.setCombinerClass(IntSumReducer.class);
job.setReducerClass(IntSumReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
While Spark is primarily associated with Scala, it runs on the JVM and has a robust Java API:
// Word count using Apache Spark's Java API
import org.apache.spark.api.java.JavaPairRDD;
import org.apache.spark.api.java.JavaRDD;
import org.apache.spark.api.java.JavaSparkContext;
import org.apache.spark.SparkConf;
import scala.Tuple2;
import java.util.Arrays;
import java.util.List;
import java.util.regex.Pattern;
public class JavaWordCount {
private static final Pattern SPACE = Pattern.compile(" ");
public static void main(String[] args) {
SparkConf sparkConf = new SparkConf().setAppName("JavaWordCount").setMaster("local[*]");
JavaSparkContext ctx = new JavaSparkContext(sparkConf);
JavaRDD<String> lines = ctx.textFile("hdfs://path/to/input");
JavaRDD<String> words = lines.flatMap(s -> Arrays.asList(SPACE.split(s)).iterator());
JavaPairRDD<String, Integer> ones = words.mapToPair(s -> new Tuple2<>(s, 1));
JavaPairRDD<String, Integer> counts = ones.reduceByKey((i1, i2) -> i1 + i2);
// Save results
counts.saveAsTextFile("hdfs://path/to/output");
ctx.stop();
}
}
Kafka, the distributed streaming platform, is built in Java and Scala:
// Producing and consuming messages with Kafka's Java API
import org.apache.kafka.clients.consumer.*;
import org.apache.kafka.clients.producer.*;
import org.apache.kafka.common.serialization.*;
import java.time.Duration;
import java.util.*;
public class KafkaExample {
public static void main(String[] args) {
// Producer configuration
Properties producerProps = new Properties();
producerProps.put("bootstrap.servers", "localhost:9092");
producerProps.put("key.serializer", StringSerializer.class.getName());
producerProps.put("value.serializer", StringSerializer.class.getName());
// Create a producer
KafkaProducer<String, String> producer = new KafkaProducer<>(producerProps);
// Send a message
producer.send(new ProducerRecord<>("sensors-data", "device-123",
"{\"temperature\":22.5,\"humidity\":45.2}"));
producer.close();
// Consumer configuration
Properties consumerProps = new Properties();
consumerProps.put("bootstrap.servers", "localhost:9092");
consumerProps.put("group.id", "temperature-monitor");
consumerProps.put("key.deserializer", StringDeserializer.class.getName());
consumerProps.put("value.deserializer", StringDeserializer.class.getName());
// Create a consumer
KafkaConsumer<String, String> consumer = new KafkaConsumer<>(consumerProps);
consumer.subscribe(Collections.singletonList("sensors-data"));
// Poll for messages
while (true) {
ConsumerRecords<String, String> records = consumer.poll(Duration.ofMillis(100));
for (ConsumerRecord<String, String> record : records) {
System.out.printf("Device: %s, Reading: %s%n",
record.key(), record.value());
}
}
}
}
Flink, a stream processing framework, leverages Java for its core functionality:
// Real-time analytics with Apache Flink
import org.apache.flink.api.common.functions.MapFunction;
import org.apache.flink.api.java.tuple.Tuple2;
import org.apache.flink.streaming.api.datastream.DataStream;
import org.apache.flink.streaming.api.environment.StreamExecutionEnvironment;
import org.apache.flink.streaming.api.windowing.time.Time;
public class SensorAnalytics {
public static void main(String[] args) throws Exception {
// Set up the streaming execution environment
final StreamExecutionEnvironment env = StreamExecutionEnvironment.getExecutionEnvironment();
// Read sensor data stream
DataStream<String> sensorStream = env.socketTextStream("localhost", 9999);
// Parse and process the data
DataStream<Tuple2<String, Double>> parsedStream = sensorStream
.map(new MapFunction<String, Tuple2<String, Double>>() {
@Override
public Tuple2<String, Double> map(String value) throws Exception {
String[] parts = value.split(",");
return new Tuple2<>(parts[0], Double.parseDouble(parts[1]));
}
});
// Calculate average temperature per sensor over 1 minute windows
DataStream<Tuple2<String, Double>> avgTemperatures = parsedStream
.keyBy(value -> value.f0) // Key by sensor ID
.timeWindow(Time.minutes(1))
.aggregate(new AverageAggregate());
// Alert on high temperatures
DataStream<Tuple2<String, Double>> alerts = avgTemperatures
.filter(value -> value.f1 > 30.0);
// Print alerts
alerts.print();
// Execute the job
env.execute("Sensor Temperature Monitoring");
}
}
This popular search and analytics engine is built on Java:
// Using Elasticsearch's Java API
import org.elasticsearch.action.index.IndexRequest;
import org.elasticsearch.action.search.SearchRequest;
import org.elasticsearch.action.search.SearchResponse;
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.client.RestHighLevelClient;
import org.elasticsearch.index.query.QueryBuilders;
import org.elasticsearch.search.builder.SearchSourceBuilder;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
public class ElasticsearchExample {
public static void main(String[] args) throws IOException {
// Create a client
RestHighLevelClient client = createClient();
// Index a document
Map<String, Object> document = new HashMap<>();
document.put("title", "Big Data Processing with Java");
document.put("author", "Jane Developer");
document.put("category", "Programming");
document.put("published_date", "2023-04-15");
IndexRequest indexRequest = new IndexRequest("articles")
.id("1001")
.source(document);
client.index(indexRequest, RequestOptions.DEFAULT);
// Search for documents
SearchRequest searchRequest = new SearchRequest("articles");
SearchSourceBuilder searchSourceBuilder = new SearchSourceBuilder();
searchSourceBuilder.query(QueryBuilders.matchQuery("category", "Programming"));
searchRequest.source(searchSourceBuilder);
SearchResponse searchResponse = client.search(searchRequest, RequestOptions.DEFAULT);
// Process results
searchResponse.getHits().forEach(hit -> {
Map<String, Object> sourceAsMap = hit.getSourceAsMap();
System.out.println("Found: " + sourceAsMap.get("title"));
});
// Close the client
client.close();
}
private static RestHighLevelClient createClient() {
// Client creation logic
return new RestHighLevelClient(/* configuration */);
}
}
Java’s long history has created a vast ecosystem of libraries, tools, and frameworks. For big data applications, this means:
- Comprehensive data structures and algorithms
- Optimized I/O and network operations
- Robust testing frameworks
- Mature build and dependency management tools
Most large enterprises already have significant Java investments, making it easier to integrate big data solutions:
// Integrating big data with enterprise systems
@RestController
@RequestMapping("/api/analytics")
public class AnalyticsController {
private final KafkaTemplate<String, String> kafkaTemplate;
private final HadoopJobService hadoopService;
private final DataLakeRepository dataLakeRepo;
// Constructor injection
@PostMapping("/events")
public ResponseEntity<String> captureEvent(@RequestBody EventData event) {
// Validate enterprise authentication
if (!securityService.isValidToken(event.getToken())) {
return ResponseEntity.status(HttpStatus.UNAUTHORIZED).build();
}
// Send to Kafka for real-time processing
kafkaTemplate.send("user-events", event.getUserId(),
objectMapper.writeValueAsString(event));
return ResponseEntity.accepted().build();
}
@GetMapping("/reports/{reportId}")
public ResponseEntity<ReportResult> getReport(@PathVariable String reportId) {
// Check if report is already generated
Optional<ReportResult> cachedReport = reportCache.findById(reportId);
if (cachedReport.isPresent()) {
return ResponseEntity.ok(cachedReport.get());
}
// Submit a Hadoop job to generate the report
String jobId = hadoopService.submitReportJob(reportId);
// Return job ID for polling
return ResponseEntity
.accepted()
.header("Job-ID", jobId)
.build();
}
}
Java remains one of the most widely taught and used programming languages, making it easier to find developers who can work on big data applications.
Java’s strong commitment to backward compatibility means big data systems can evolve without breaking existing functionality:
// Example of how Java maintains backward compatibility
// Old code from 2010
public class LegacyDataProcessor {
public List<CustomerRecord> processRecords(List<String> rawRecords) {
List<CustomerRecord> result = new ArrayList<CustomerRecord>();
for (String record : rawRecords) {
// Process each record
result.add(parseRecord(record));
}
return result;
}
private CustomerRecord parseRecord(String record) {
// Legacy parsing logic
return new CustomerRecord(record.split(","));
}
}
// Modern code from 2023 that can still work with the legacy component
public class ModernDataPipeline {
private final LegacyDataProcessor legacyProcessor;
public ModernDataPipeline(LegacyDataProcessor legacyProcessor) {
this.legacyProcessor = legacyProcessor;
}
public CompletableFuture<List<EnrichedCustomerRecord>> processDataAsync(
Stream<String> dataStream) {
// Convert stream to list for legacy component
List<String> rawRecords = dataStream.collect(Collectors.toList());
// Use the legacy processor
return CompletableFuture.supplyAsync(() -> {
List<CustomerRecord> processedRecords = legacyProcessor.processRecords(rawRecords);
// Enrich with modern techniques
return processedRecords.stream()
.map(this::enrichRecord)
.collect(Collectors.toList());
});
}
private EnrichedCustomerRecord enrichRecord(CustomerRecord record) {
// Modern enrichment logic
return new EnrichedCustomerRecord(record);
}
}
While Java excels for big data applications, there are some challenges to consider:
Java code can be more verbose than newer languages, which can impact development speed:
// Java's traditional verbosity
Map<String, List<TransactionRecord>> transactionsByCustomer = new HashMap<>();
for (TransactionRecord transaction : transactions) {
String customerId = transaction.getCustomerId();
if (!transactionsByCustomer.containsKey(customerId)) {
transactionsByCustomer.put(customerId, new ArrayList<>());
}
transactionsByCustomer.get(customerId).add(transaction);
}
// Modern Java (Java 8+) is more concise
Map<String, List<TransactionRecord>> modernMap = transactions.stream()
.collect(Collectors.groupingBy(TransactionRecord::getCustomerId));
The JVM has traditionally had slower startup times compared to some interpreted languages, though this is less relevant for long-running big data processes.
Java’s object model and garbage collection can introduce memory overhead compared to languages with manual memory management.
Java continues to evolve with features that benefit big data applications:
This ongoing project aims to introduce value types, which will reduce the memory overhead of Java objects—a significant benefit for data-intensive applications.
Virtual threads and structured concurrency will make it easier to write highly concurrent data processing code:
// Future Java with Project Loom (preview)
void processMillionsOfRecords(List<Record> records) {
// Each record gets its own virtual thread - can scale to millions
try (var executor = Executors.newVirtualThreadPerTaskExecutor()) {
for (Record record : records) {
executor.submit(() -> {
processRecord(record);
});
}
} // Executor is auto-closed, waits for all tasks
}
Improved native interoperability will allow Java to better interface with specialized native libraries for data processing.
// Use primitive collections for better performance
import it.unimi.dsi.fastutil.longs.Long2DoubleOpenHashMap;
public class EfficientFeatureVector {
// Instead of Map<Long, Double> which boxes primitives
private final Long2DoubleOpenHashMap features;
public EfficientFeatureVector() {
// Specialized map for primitive long->double mapping
this.features = new Long2DoubleOpenHashMap();
}
public void setFeature(long featureId, double value) {
features.put(featureId, value);
}
public double dotProduct(EfficientFeatureVector other) {
double result = 0.0;
// Iterate over the smaller vector for efficiency
if (this.features.size() < other.features.size()) {
for (Long2DoubleOpenHashMap.Entry entry : features.long2DoubleEntrySet()) {
long featureId = entry.getLongKey();
double value = entry.getDoubleValue();
result += value * other.features.getOrDefault(featureId, 0.0);
}
} else {
// Same logic but iterating over other
}
return result;
}
}
// Managing memory in data-intensive applications
public class DataProcessor {
public void processBatches(Iterator<DataBatch> batchIterator) {
while (batchIterator.hasNext()) {
DataBatch batch = batchIterator.next();
try {
processBatch(batch);
} finally {
// Explicitly clear references to help GC
batch.clear();
}
// Suggest garbage collection after processing large batches
// Note: This is just a hint to the JVM
System.gc();
}
}
private void processBatch(DataBatch batch) {
// Process in chunks to manage memory better
List<Record> records = batch.getRecords();
int chunkSize = 1000;
for (int i = 0; i < records.size(); i += chunkSize) {
int end = Math.min(i + chunkSize, records.size());
List<Record> chunk = records.subList(i, end);
processChunk(chunk);
// Help garbage collector by clearing references
chunk.clear();
}
}
private void processChunk(List<Record> chunk) {
// Actual data processing logic
}
}
// Using CompletableFuture for asynchronous operations
public class AsyncDataProcessor {
private final ExecutorService executor;
public AsyncDataProcessor(int threadPoolSize) {
this.executor = Executors.newFixedThreadPool(threadPoolSize);
}
public CompletableFuture<ProcessingResult> processDataAsync(List<DataItem> items) {
// Split into chunks for parallel processing
int chunkSize = items.size() / Runtime.getRuntime().availableProcessors();
chunkSize = Math.max(chunkSize, 100); // Minimum chunk size
List<List<DataItem>> chunks = splitIntoChunks(items, chunkSize);
// Process each chunk asynchronously
List<CompletableFuture<ChunkResult>> chunkFutures = chunks.stream()
.map(chunk -> CompletableFuture.supplyAsync(
() -> processChunk(chunk), executor))
.collect(Collectors.toList());
// Combine all results when complete
return CompletableFuture.allOf(
chunkFutures.toArray(new CompletableFuture[0]))
.thenApply(v -> {
List<ChunkResult> results = chunkFutures.stream()
.map(CompletableFuture::join)
.collect(Collectors.toList());
return combineResults(results);
});
}
private List<List<DataItem>> splitIntoChunks(List<DataItem> items, int chunkSize) {
// Implementation to split list into chunks
}
private ChunkResult processChunk(List<DataItem> chunk) {
// Process a single chunk
}
private ProcessingResult combineResults(List<ChunkResult> results) {
// Combine chunk results
}
public void shutdown() {
executor.shutdown();
try {
if (!executor.awaitTermination(30, TimeUnit.SECONDS)) {
executor.shutdownNow();
}
} catch (InterruptedException e) {
executor.shutdownNow();
Thread.currentThread().interrupt();
}
}
}
Java’s presence in the big data ecosystem is no accident. Its combination of performance, reliability, and platform independence made it the natural choice as the foundation for many of the tools and frameworks that power today’s data-driven world.
As we move into the era of AI, edge computing, and ever-increasing data volumes, Java continues to adapt and evolve. Its strong backwards compatibility ensures that the massive investment in Java-based big data infrastructure remains valuable, while new language features address emerging challenges.
For developers looking to work in big data, learning Java provides access to a vast ecosystem of technologies and opportunities. While newer languages may grab headlines, Java quietly continues to run the systems that process, analyze, and derive value from the world’s data.
The next time you analyze a dataset with Spark, search with Elasticsearch, or process a stream with Kafka, remember that Java is working behind the scenes, demonstrating why it has remained relevant for over 25 years and why it will likely continue to be a cornerstone of big data technologies for years to come.
#Java #BigData #DataEngineering #Hadoop #ApacheSpark #Kafka #JVM #Programming #SoftwareDevelopment #DataProcessing #DistributedSystems #CloudComputing #DataScience #JavaProgramming #ApacheFlink #Elasticsearch #DataArchitecture #EnterpriseSoftware #StreamProcessing #ProgrammingLanguages