Apache Airflow: Mastering Workflow Orchestration for Modern Data Engineering
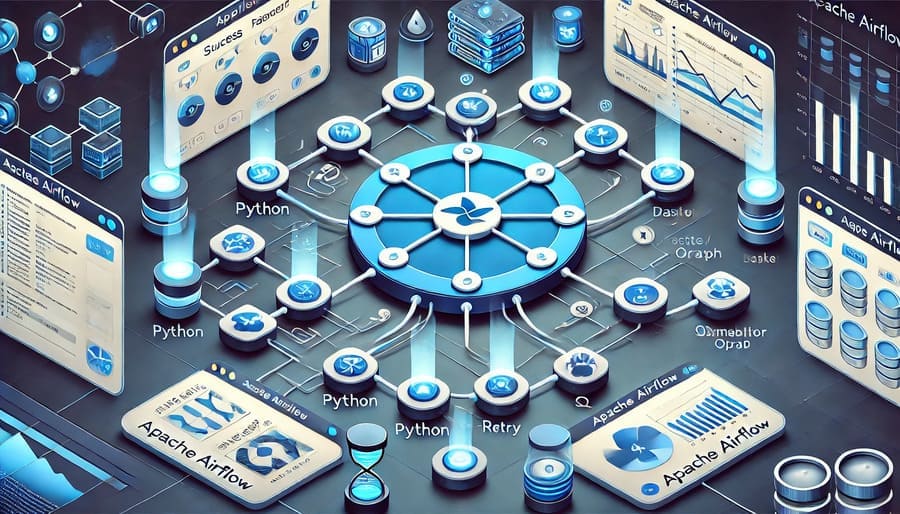
In the complex world of data engineering, managing interdependent tasks seamlessly is critical for success. Enter Apache Airflow—an open-source platform that has revolutionized how organizations author, schedule, and monitor workflows. As a cornerstone technology in the data engineering toolkit, Airflow offers powerful capabilities that transform chaotic data processes into well-orchestrated, observable, and reliable pipelines.
Airflow was born at Airbnb in 2014 when Maxime Beauchemin, facing the challenges of managing increasingly complex data workflows, created a solution that would change the data engineering landscape forever. The project was later donated to the Apache Software Foundation, where it has flourished with contributions from thousands of developers worldwide.
The core philosophy behind Airflow is simple yet powerful: workflows should be defined as code. This programmatic approach brings all the benefits of software development practices to workflow management—version control, testing, modularity, and collaboration.
At the heart of Airflow lies the concept of DAGs—Directed Acyclic Graphs. DAGs represent workflows as collections of tasks with directional dependencies. The “acyclic” nature ensures that workflows never create circular dependencies, preventing infinite loops.
DAGs in Airflow are defined using Python code, providing flexibility and expressiveness far beyond what’s possible with configuration-based alternatives. A simple example illustrates this elegance:
from datetime import datetime, timedelta
from airflow import DAG
from airflow.operators.python import PythonOperator
default_args = {
'owner': 'data_engineer',
'depends_on_past': False,
'start_date': datetime(2023, 1, 1),
'email_on_failure': False,
'email_on_retry': False,
'retries': 1,
'retry_delay': timedelta(minutes=5),
}
def extract_data():
# Data extraction logic
return {"extracted_data": "sample"}
def transform_data(ti):
extracted_data = ti.xcom_pull(task_ids=['extract_data'])[0]
# Transformation logic
return {"transformed_data": "processed"}
def load_data(ti):
transformed_data = ti.xcom_pull(task_ids=['transform_data'])[0]
# Loading logic
return "Data loaded successfully"
with DAG(
'simple_etl_pipeline',
default_args=default_args,
schedule_interval='@daily',
catchup=False,
) as dag:
extract_task = PythonOperator(
task_id='extract_data',
python_callable=extract_data,
)
transform_task = PythonOperator(
task_id='transform_data',
python_callable=transform_data,
)
load_task = PythonOperator(
task_id='load_data',
python_callable=load_data,
)
extract_task >> transform_task >> load_task
Operators define what gets done, encapsulating the logic for a single task in a workflow. Airflow provides a rich set of built-in operators:
- PythonOperator: Executes Python functions
- BashOperator: Executes bash commands
- SQLOperator: Executes SQL queries
- EmailOperator: Sends emails
- S3ToRedshiftOperator: Transfers data from Amazon S3 to Redshift
The ecosystem extends further with provider packages for virtually every data platform and service, from AWS and Google Cloud to Snowflake, Databricks, and beyond.
When a DAG runs, each task becomes a Task Instance—a specific execution of that task at a point in time. These instances track state (running, success, failed), retry information, and execution duration, providing crucial observability.
XComs enable tasks to exchange small amounts of data. While not designed for transferring large datasets, they’re perfect for passing metadata, status information, or configuration between dependent tasks.
Airflow’s architecture consists of several components working together:
- Web Server: Provides the user interface for monitoring and managing DAGs
- Scheduler: Determines which tasks need to be executed when
- Workers: Execute the tasks assigned by the scheduler
- Metadata Database: Stores state information about DAGs and tasks
- DAG Directory: Contains the Python files defining workflows
This modular architecture allows Airflow to scale from single-server deployments to massive distributed environments handling thousands of concurrent tasks.
Several factors have contributed to Airflow’s widespread adoption:
The decision to use Python for workflow definition provides tremendous advantages:
- Access to Python’s rich ecosystem of libraries
- Ability to implement complex conditional logic
- Dynamic DAG generation based on external configurations
- Testing workflows using standard Python testing frameworks
Airflow’s web interface provides comprehensive visibility into workflow execution:
- DAG visualization showing task dependencies
- Detailed execution logs for debugging
- Historical run data for performance analysis
- Easy manual triggering of tasks or DAGs
The plugin system allows organizations to extend Airflow with:
- Custom operators for specific technologies
- Hooks for connecting to external systems
- Sensors for waiting on external conditions
- Custom views in the web interface
Beyond simple time-based scheduling, Airflow supports:
- Data-driven triggers (sensors)
- Event-based execution
- Backfilling historical runs
- Catchup for handling gaps in execution
Successful Airflow implementations typically follow these guidelines:
Design tasks to be idempotent—they should produce the same results regardless of how many times they’re executed. This property is crucial for recovery from failures.
Use sensors wisely to wait for conditions like file arrivals or external process completion, but be cautious of blocking worker slots with long-running sensors.
Leverage Airflow’s parameterization capabilities to make DAGs reusable across different datasets, timeframes, or environments.
# Parameterized DAG example
with DAG(
'process_data_{{ params.data_source }}',
params={'data_source': 'default', 'processing_type': 'full'},
# Other DAG parameters
) as dag:
# Tasks can access params via {{ params.data_source }}
Implement common patterns like:
- Fan-out/fan-in: Parallelize processing across datasets, then aggregate results
- Staging and promotion: Process data in staging areas before promoting to production
- Dynamic task generation: Create tasks based on runtime discovery
Set up comprehensive monitoring by:
- Configuring failure notifications
- Integrating with monitoring platforms
- Setting SLAs for critical tasks
- Tracking DAG performance metrics
Organizations can deploy Airflow in several ways:
- On-premises infrastructure
- Cloud virtual machines
- Kubernetes using the official Helm chart
- Amazon MWAA (Managed Workflows for Apache Airflow)
- Google Cloud Composer
- Astronomer
- Azure Data Factory with Airflow integration
Airflow continues to evolve with significant improvements:
Major enhancements in recent versions include:
- A lightweight, fast task execution framework
- Simplified REST API
- Improved scheduler performance
- Full support for custom timetables
- Enhanced security features
Airflow increasingly integrates with complementary tools:
- dbt: For data transformation
- Great Expectations: For data quality
- Dagster: For data-aware orchestration
- Prefect: For dynamic workflow patterns
Apache Airflow excels in various scenarios:
Orchestrating the extraction, transformation, and loading of data between systems, particularly with complex dependencies.
Managing the end-to-end ML lifecycle from data preparation to model training, evaluation, and deployment.
Scheduling and monitoring data validation jobs across data warehouses and lakes.
Automating the creation and distribution of periodic reports and dashboards.
Despite its strengths, Airflow has challenges to consider:
- Learning curve for new users
- Resource requirements for large-scale deployments
- Overhead for very simple workflows
- Historical limitations with dynamic task generation (improving in newer versions)
Apache Airflow has established itself as the leading workflow orchestration platform for data engineering. Its combination of programmatic flexibility, robust scheduling, comprehensive monitoring, and active community makes it an essential tool for organizations building complex data pipelines.
As data ecosystems grow increasingly complex, Airflow’s ability to bring order to chaos through code-defined workflows gives data engineers the power to build reliable, observable, and maintainable data processes. Whether you’re just starting with data orchestration or looking to optimize existing workflows, Apache Airflow provides a solid foundation for your data engineering infrastructure.
Keywords: Apache Airflow, workflow orchestration, DAGs, data pipeline, task scheduling, data engineering, ETL automation, workflow management, directed acyclic graphs, Python workflows
Hashtags: #ApacheAirflow #DataEngineering #WorkflowOrchestration #DAG #DataPipelines #ETL #PythonAutomation #DataOps #AirflowScheduling #OpenSourceData