Luigi: Mastering Pipeline Orchestration with Python’s Powerful Workflow Engine
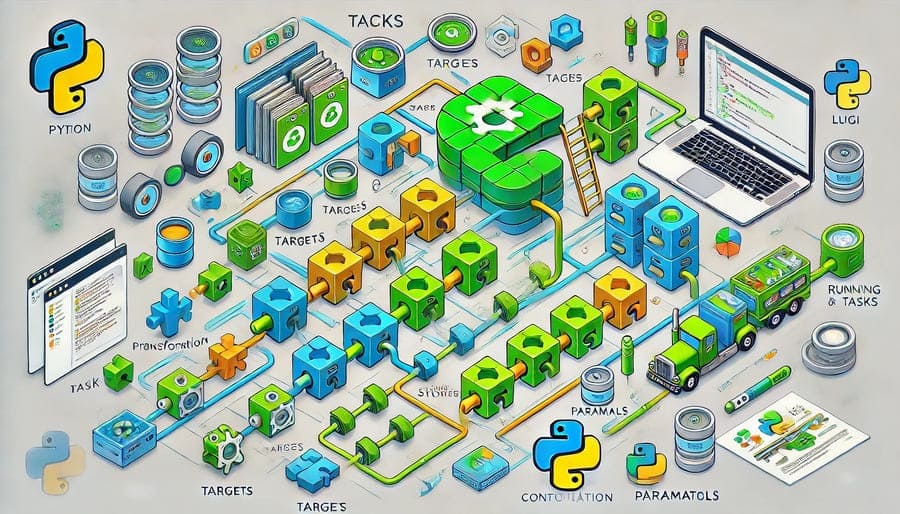
In the ever-expanding universe of data engineering, managing complex data workflows efficiently is a constant challenge. Enter Luigi—a powerful Python framework developed by Spotify that has transformed how data professionals build, manage, and monitor data pipelines. Named after the famous video game character, Luigi helps engineers tackle the monstrous task of workflow management with elegance and simplicity.
Luigi was born out of necessity at Spotify, where engineers faced the daunting challenge of orchestrating complex data processing workflows across their massive music streaming platform. In 2012, Spotify open-sourced Luigi, sharing their solution with the wider data community. Since then, the framework has gained significant adoption across industries, from finance and healthcare to e-commerce and social media.
At its heart, Luigi embodies a straightforward yet powerful approach to workflow management based on a few key principles:
- Task-based architecture: Everything in Luigi revolves around tasks—self-contained units of work with clearly defined inputs and outputs.
- Dependency resolution: Luigi excels at managing complex dependency chains, ensuring tasks run only when their prerequisites are complete.
- Failure recovery: When something goes wrong (and it inevitably will), Luigi provides mechanisms to recover gracefully without rerunning successful tasks.
- Visualization: Luigi’s built-in web interface offers real-time insights into pipeline execution, making complex workflows understandable at a glance.
Tasks represent the fundamental unit of work in Luigi. Each task typically follows this pattern:
import luigi
class ProcessLogs(luigi.Task):
date = luigi.DateParameter()
def requires(self):
return FetchLogs(self.date)
def output(self):
return luigi.LocalTarget(f"processed_logs_{self.date}.csv")
def run(self):
# Processing logic here
with self.input().open('r') as infile, self.output().open('w') as outfile:
# Transform data from infile to outfile
for line in infile:
processed_line = line.strip().upper()
outfile.write(processed_line + '\n')
Every Luigi task implements three key methods:
- requires(): Specifies dependencies—other tasks that must complete before this one starts
- output(): Defines what the task produces, typically files or database records
- run(): Contains the actual processing logic to execute
Targets represent the output of tasks, providing abstraction over different storage systems:
- LocalTarget: For files on the local filesystem
- S3Target: For files in Amazon S3
- MySqlTarget: For MySQL database tables
- HdfsTarget: For files in Hadoop Distributed File System
- RedshiftTarget: For Amazon Redshift tables
Custom targets can be created to support virtually any storage system.
Parameters make tasks configurable and reusable across different datasets or time periods:
class GenerateReport(luigi.Task):
date = luigi.DateParameter()
region = luigi.Parameter(default="global")
include_metrics = luigi.ListParameter(default=["revenue", "users"])
email_report = luigi.BoolParameter(default=False)
These parameters can be passed when triggering workflows, enabling dynamic pipeline behavior.
class ExtractData(luigi.Task):
date = luigi.DateParameter()
def output(self):
return luigi.LocalTarget(f"data/raw_{self.date}.json")
def run(self):
# Extract data from source
with self.output().open('w') as outfile:
# Write extracted data
pass
class TransformData(luigi.Task):
date = luigi.DateParameter()
def requires(self):
return ExtractData(self.date)
def output(self):
return luigi.LocalTarget(f"data/transformed_{self.date}.csv")
def run(self):
# Transform logic
with self.input().open('r') as infile, self.output().open('w') as outfile:
# Transform data
pass
class LoadData(luigi.Task):
date = luigi.DateParameter()
def requires(self):
return TransformData(self.date)
def output(self):
return luigi.LocalTarget(f"data/loaded_{self.date}.success")
def run(self):
# Load data to destination
with self.input().open('r') as infile:
# Load data
with self.output().open('w') as outfile:
outfile.write("Success!")
class ETLPipeline(luigi.WrapperTask):
date = luigi.DateParameter()
def requires(self):
return LoadData(self.date)
This example demonstrates a straightforward ETL pipeline with extract, transform, and load stages, wrapped in a single task for easy triggering.
class PrepareFeatures(luigi.Task):
date = luigi.DateParameter()
def output(self):
return luigi.LocalTarget(f"data/features_{self.date}.csv")
def run(self):
# Feature engineering
pass
class TrainModel(luigi.Task):
date = luigi.DateParameter()
model_type = luigi.Parameter(default="random_forest")
def requires(self):
return PrepareFeatures(self.date)
def output(self):
return luigi.LocalTarget(f"models/{self.model_type}_{self.date}.pkl")
def run(self):
# Model training logic
pass
class EvaluateModel(luigi.Task):
date = luigi.DateParameter()
model_type = luigi.Parameter(default="random_forest")
def requires(self):
return TrainModel(date=self.date, model_type=self.model_type)
def output(self):
return luigi.LocalTarget(f"metrics/{self.model_type}_{self.date}.json")
def run(self):
# Model evaluation
pass
This workflow handles the typical machine learning pipeline steps: feature preparation, model training, and evaluation.
- Simplicity: Luigi typically has a gentler learning curve than Airflow.
- Python-centric: Luigi is deeply integrated with Python, making it natural for Python developers.
- File-based dependencies: Luigi excels at tracking dependencies through files, while Airflow has more abstract dependency management.
- Scheduling: Airflow has more robust scheduling capabilities built-in.
- Maturity: Luigi has been battle-tested in production for many years.
- Modern features: Prefect introduces more modern features like dynamic workflows.
- Developer experience: Prefect generally offers a more polished developer experience.
- Data-aware orchestration: Dagster is more “data-aware” with type checking and data contracts.
- Testing: Dagster provides better testing capabilities for workflows.
- Simplicity: Luigi maintains a simpler model that can be easier to get started with.
For complex workflows:
- Organize tasks in modules based on their domain
- Use wrapper tasks to group logical units
- Leverage inheritance for common task patterns
- Create reusable mixins for cross-cutting concerns
Robust error management is crucial:
def run(self):
try:
# Processing logic
with self.input().open('r') as infile:
data = json.load(infile)
# Process data
processed_data = self._transform_data(data)
with self.output().open('w') as outfile:
json.dump(processed_data, outfile)
except Exception as e:
logger.error(f"Task failed: {str(e)}")
raise
- Take advantage of Luigi’s built-in visualization
- Implement custom event handlers for notifications
- Add logging throughout task execution
- Consider integrating with monitoring platforms like Prometheus
class MyTask(luigi.Task):
def run(self):
logger.info("Starting task execution")
start_time = time.time()
# Task logic
execution_time = time.time() - start_time
logger.info(f"Task completed in {execution_time:.2f} seconds")
Effective testing ensures reliable pipelines:
class TestExtractTask(unittest.TestCase):
def setUp(self):
# Setup test environment
pass
def test_extraction(self):
# Create a test instance
task = ExtractData(date=datetime.date.today())
# Run the task
luigi.build([task], local_scheduler=True)
# Assert output exists and has correct content
self.assertTrue(task.output().exists())
with task.output().open('r') as f:
data = json.load(f)
self.assertIn('expected_field', data)
Luigi can be executed in various ways:
- Command line:
luigi --module my_tasks MyTask --param1 value1
- Python API:
luigi.build([MyTask(param1="value1")], workers=3)
- Scheduled jobs: Using cron, systemd, or other schedulers
For larger workloads:
- Use the central scheduler for coordinating multiple workers
- Implement task prioritization for critical path optimization
- Consider containerization with Docker for consistent environments
- Leverage Kubernetes for orchestrating Luigi workers
# Start the central scheduler
luigid --background
# Run workers pointing to the central scheduler
luigi --module my_tasks MyTask --scheduler-host luigi-scheduler.example.com
While newer orchestration tools have emerged, Luigi maintains a strong position in the data engineering ecosystem thanks to its:
- Battle-tested reliability
- Simplicity and clarity of concept
- Strong integration with Python
- Active community support
- Continued development and maintenance
Recent improvements focus on better integration with cloud services, enhanced visualization, and performance optimizations.
Luigi remains a powerful choice for data engineers seeking a pythonic, file-centric approach to workflow orchestration. Its focus on simplicity, dependency management, and failure recovery makes it particularly well-suited for data transformation tasks, ETL pipelines, and machine learning workflows.
Whether you’re building a small analytical pipeline or orchestrating complex data workflows across distributed systems, Luigi provides the building blocks needed to create robust, maintainable data orchestration solutions. By focusing on the fundamental primitives of tasks, dependencies, and targets, Luigi empowers engineers to tackle complex problems with elegance and clarity.
As the data orchestration landscape continues to evolve, Luigi’s straightforward approach and deep Python integration ensure it remains a valuable tool in the modern data engineer’s toolkit.
Keywords: Luigi Python, workflow orchestration, data pipeline, task dependencies, ETL pipeline, Luigi framework, Python automation, Spotify engineering, data workflow, pipeline management
Hashtags: #LuigiPython #DataPipelines #WorkflowOrchestration #PythonAutomation #ETLFramework #DataEngineering #TaskOrchestration #OpenSource #DataWorkflow #PythonFramework