SCD Type 3: Add New Attributes – The Elegant Approach to Limited Historical Tracking
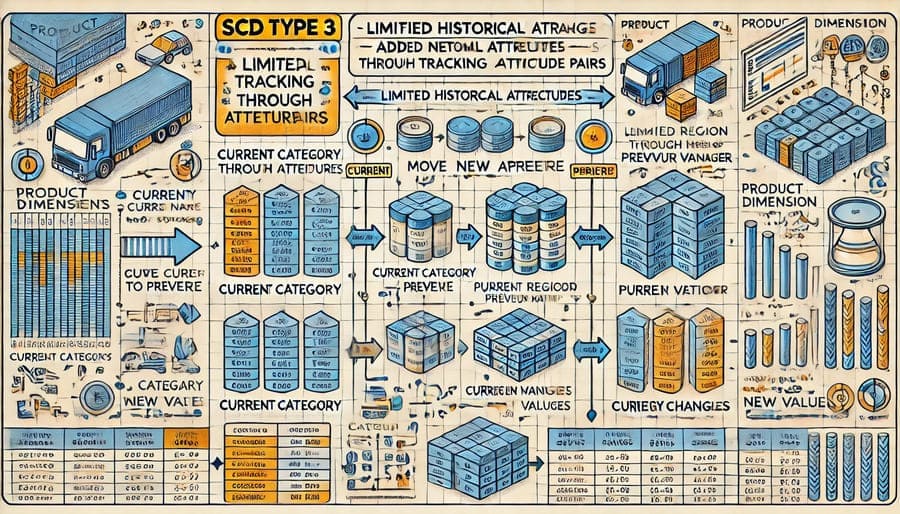
In the world of data warehousing, managing dimensional changes effectively is crucial for maintaining analytical accuracy while balancing performance and complexity. Among the various Slowly Changing Dimension (SCD) methodologies, Type 3 offers a unique approach that sits between the simplicity of Type 1 and the comprehensive historical tracking of Type 2. By adding new attributes to store previous values alongside current ones, SCD Type 3 provides an elegant solution for scenarios where limited historical context is valuable without the overhead of full versioning.
At its core, SCD Type 3 follows a distinctive approach: when attribute values change, retain both the current and previous values in separate columns within the same row. This technique creates a dimensional structure that simultaneously presents both current reality and a single historical state, enabling straightforward “before and after” analysis.
The defining characteristics that make Type 3 unique in the SCD taxonomy include:
- Limited Historical Preservation: Typically stores just one previous state (sometimes more)
- Expanded Column Structure: Adds new columns to hold historical values
- Single-Row Representation: Maintains one row per business entity
- Attribute-Paired Design: Creates current/previous pairs for tracked attributes
- Change Tracking Metadata: Often includes dates or indicators of when values changed
A standard Type 3 dimension includes explicit columns for both current and previous values:
DimProduct {
ProductKey (PK)
ProductID (Natural Key)
// Current values
CurrentProductName
CurrentCategory
CurrentSubcategory
CurrentDepartment
// Previous values
PreviousProductName
PreviousCategory
PreviousSubcategory
PreviousDepartment
// Change tracking metadata
CategoryChangeDate
DepartmentChangeDate
// Regular attributes (not tracked historically)
Brand
Size
Color
UnitPrice
Status
}
In this structure, each product has a single row containing both its current classification and its previous classification, enabling straightforward comparison between the two states.
SCD Type 3 shines in specific scenarios where its balanced approach to historical tracking delivers optimal value:
When direct comparison between current and previous states is central to analysis:
- Marketing Campaign Impact: Comparing metrics before and after category changes
- Reorganization Effects: Analyzing performance across organizational restructuring
- Pricing Strategy Evaluation: Measuring results before and after price tier adjustments
- Classification Shift Analysis: Understanding the impact of entity reclassification
When significant, planned changes occur across the organization:
- Corporate Reorganizations: Tracking departmental shifts during restructuring
- Product Line Reclassifications: Managing category realignment initiatives
- Territory Redistribution: Analyzing customer reassignment to new territories
- Seasonal Catalog Revisions: Handling seasonal category adjustments
When some history is valuable but full versioning is impractical:
- Mobile Applications: Where storage efficiency matters
- Edge Computing Scenarios: With limited storage capacity
- High-Cardinality Dimensions: Containing millions of members
- Performance-Critical Systems: Where query simplicity is essential
When processes inherently involve precisely two states:
- Fiscal Year Transitions: Comparing current and previous fiscal structures
- Annual Budget Cycles: Analyzing current budget categories vs. previous year
- Biennial Reviews: Comparing current and previous review periods
- Contract Renewals: Examining current vs. previous contract terms
Implementing SCD Type 3 requires specific technical approaches to ensure both current and historical values are maintained correctly.
The fundamental structure must support both current and previous states:
Creating matched sets of columns for tracked attributes:
- Current/previous naming convention
- Consistent data types between pairs
- Nullable previous value columns
- Clear column organization in schema
Tracking when and why changes occurred:
- ChangeDate: When the attribute was updated
- ChangedBy: Who or what system made the change
- ChangeReason: Categorization of change drivers
- PreviousValueCount: How many changes have occurred
For advanced Type 3 implementations:
- Version-Specific Columns: Current, Previous1, Previous2
- Array Structures: JSON or array columns in modern databases
- XML Attributes: Structured historical data in XML columns
- Vertical History Tables: Companion tables for deeper history
The process of maintaining Type 3 dimensions requires careful attribute management:
During the first load of a dimension:
- Populate current value columns with source data
- Leave previous value columns NULL
- Set change dates to NULL or load date
- Load other non-historical attributes normally
When updating the dimension:
- Identify records with changes to tracked attributes
- For changed attributes, move current values to previous columns
- Update current columns with new values
- Set change dates to current date (or business effective date)
- Update non-tracked attributes directly
-- Step 1: Identify records with category changes
CREATE TABLE #ChangedProducts AS
SELECT
S.ProductID,
T.ProductKey,
T.CurrentCategory AS OldCategory,
S.Category AS NewCategory,
T.CategoryChangeDate
FROM StageProduct S
JOIN DimProduct T ON S.ProductID = T.ProductID
WHERE S.Category <> T.CurrentCategory;
-- Step 2: Update dimension with changes
UPDATE DimProduct
SET
PreviousCategory = CurrentCategory,
CurrentCategory = S.Category,
CategoryChangeDate = GETDATE()
FROM DimProduct P
JOIN #ChangedProducts C ON P.ProductKey = C.ProductKey
JOIN StageProduct S ON P.ProductID = S.ProductID;
-- Step 3: Update non-tracked attributes
UPDATE DimProduct
SET
Brand = S.Brand,
Size = S.Size,
Color = S.Color,
UnitPrice = S.UnitPrice,
Status = S.Status
FROM DimProduct P
JOIN StageProduct S ON P.ProductID = S.ProductID
WHERE
P.Brand <> S.Brand OR
P.Size <> S.Size OR
P.Color <> S.Color OR
P.UnitPrice <> S.UnitPrice OR
P.Status <> S.Status;
-- Step 4: Insert new products
INSERT INTO DimProduct (
ProductID,
CurrentProductName,
CurrentCategory,
CurrentSubcategory,
CurrentDepartment,
PreviousProductName,
PreviousCategory,
PreviousSubcategory,
PreviousDepartment,
CategoryChangeDate,
DepartmentChangeDate,
Brand,
Size,
Color,
UnitPrice,
Status
)
SELECT
S.ProductID,
S.ProductName,
S.Category,
S.Subcategory,
S.Department,
NULL, -- No previous name yet
NULL, -- No previous category yet
NULL, -- No previous subcategory yet
NULL, -- No previous department yet
NULL, -- No category changes yet
NULL, -- No department changes yet
S.Brand,
S.Size,
S.Color,
S.UnitPrice,
S.Status
FROM StageProduct S
WHERE NOT EXISTS (
SELECT 1 FROM DimProduct P
WHERE P.ProductID = S.ProductID
);
In practice, Type 3 tracking is typically applied to a subset of attributes:
-- Selectively applying Type 3 to only category and department
UPDATE DimProduct
SET
-- Type 3 attributes
PreviousCategory = CASE
WHEN CurrentCategory <> S.Category
THEN CurrentCategory
ELSE PreviousCategory
END,
CurrentCategory = S.Category,
CategoryChangeDate = CASE
WHEN CurrentCategory <> S.Category
THEN GETDATE()
ELSE CategoryChangeDate
END,
PreviousDepartment = CASE
WHEN CurrentDepartment <> S.Department
THEN CurrentDepartment
ELSE PreviousDepartment
END,
CurrentDepartment = S.Department,
DepartmentChangeDate = CASE
WHEN CurrentDepartment <> S.Department
THEN GETDATE()
ELSE DepartmentChangeDate
END,
-- Type 1 attributes (simple overwrites)
Brand = S.Brand,
Size = S.Size,
Color = S.Color,
UnitPrice = S.UnitPrice,
Status = S.Status
FROM DimProduct P
JOIN StageProduct S ON P.ProductID = S.ProductID;
The power of Type 3 dimensions comes from their ability to facilitate straightforward comparative analysis.
Retrieving only the current values:
-- Simple current state query
SELECT
ProductID,
CurrentProductName,
CurrentCategory,
CurrentSubcategory,
CurrentDepartment
FROM DimProduct;
Examining the previous values:
-- Products with their previous categories
SELECT
ProductID,
CurrentProductName,
CurrentCategory,
PreviousCategory,
CategoryChangeDate
FROM DimProduct
WHERE PreviousCategory IS NOT NULL;
The true strength of Type 3 – direct before and after comparison:
-- Sales comparison before and after category change
WITH ProductShifts AS (
SELECT
P.ProductKey,
P.ProductID,
P.CurrentProductName,
P.CurrentCategory AS NewCategory,
P.PreviousCategory AS OldCategory,
P.CategoryChangeDate
FROM DimProduct P
WHERE P.PreviousCategory IS NOT NULL
)
SELECT
PS.ProductID,
PS.CurrentProductName,
PS.OldCategory,
PS.NewCategory,
SUM(CASE WHEN S.OrderDate < PS.CategoryChangeDate THEN S.SalesAmount ELSE 0 END) AS SalesBeforeChange,
SUM(CASE WHEN S.OrderDate >= PS.CategoryChangeDate THEN S.SalesAmount ELSE 0 END) AS SalesAfterChange,
COUNT(DISTINCT CASE WHEN S.OrderDate < PS.CategoryChangeDate THEN S.CustomerKey ELSE NULL END) AS CustomersBeforeChange,
COUNT(DISTINCT CASE WHEN S.OrderDate >= PS.CategoryChangeDate THEN S.CustomerKey ELSE NULL END) AS CustomersAfterChange
FROM ProductShifts PS
JOIN FactSales S ON PS.ProductKey = S.ProductKey
GROUP BY
PS.ProductID,
PS.CurrentProductName,
PS.OldCategory,
PS.NewCategory;
Examining patterns in how attributes change:
-- Category transition analysis
SELECT
PreviousCategory,
CurrentCategory,
COUNT(*) AS TransitionCount,
AVG(DATEDIFF(day, CategoryChangeDate, GETDATE())) AS AvgDaysSinceTransition
FROM DimProduct
WHERE PreviousCategory IS NOT NULL
GROUP BY PreviousCategory, CurrentCategory
ORDER BY COUNT(*) DESC;
To fully appreciate Type 3, it’s valuable to contrast it with other SCD methodologies:
Aspect | Type 3 (Add New Attribute) | Type 1 (Overwrite) |
---|---|---|
Value Changes | Keep current + previous | Overwrite with new values |
Historical Data | Limited history (previous value) | No history |
Query Complexity | Simple | Simple |
Storage Impact | Moderate (fixed columns) | Minimal |
Implementation Complexity | Moderate | Low |
Use Case | Before/after comparison needed | Current state only matters |
Aspect | Type 3 (Add New Attribute) | Type 2 (Add New Row) |
---|---|---|
Value Changes | Keep current + previous | New row with new values |
Historical Data | Limited history (previous value) | Complete history |
Query Complexity | Simple | Moderate |
Storage Impact | Moderate (fixed columns) | Grows with change frequency |
Implementation Complexity | Moderate | Moderate to high |
Use Case | Before/after comparison needed | Full historical tracking needed |
Aspect | Type 3 (Add New Attribute) | Type 6 (Hybrid) |
---|---|---|
Value Changes | Keep current + previous | Complex approach combining Types 1, 2, and 3 |
Historical Data | Limited history (previous value) | Complete history + current flags + previous values |
Query Complexity | Simple | Complex but flexible |
Storage Impact | Moderate (fixed columns) | Very significant |
Implementation Complexity | Moderate | High |
Use Case | Before/after comparison needed | Maximum flexibility needed |
In practice, dimensions often implement mixed SCD types for different attributes:
A common pattern for balanced current state and limited history:
ProductDimension {
ProductKey (PK)
ProductID
// Type 3 attributes
CurrentCategory
PreviousCategory
CategoryChangeDate
// Type 1 attributes
ProductName
Brand
Color
Size
UnitPrice
Status
}
This approach provides historical context for important classification attributes while maintaining simplicity for descriptive attributes.
When both full history and simplified before/after analysis are needed:
CustomerDimension {
CustomerKey (PK)
CustomerID
// Core attributes (Type 2 via multiple rows)
CustomerName
CustomerAddress
CustomerSegment
// Type 3 overlay within Type 2
CurrentCreditScore
PreviousCreditScore
CreditScoreChangeDate
// Type 2 tracking
EffectiveStartDate
EffectiveEndDate
CurrentFlag
}
This sophisticated approach creates new rows for major changes while also tracking immediate previous values for specific attributes within each version.
Type 6 (the combination of Types 1, 2, and 3) leverages Type 3 for specific comparative needs:
ProductDimension {
ProductKey (PK)
ProductID
// Type 2 tracking (via multiple rows)
EffectiveStartDate
EffectiveEndDate
CurrentFlag
// Type 1 current values
CurrentProductName
CurrentCategory
// Type 3 previous values
PreviousCategory
CategoryChangeDate
// Regular attributes
Brand
Size
Color
}
This comprehensive approach provides maximum analytical flexibility, though at the cost of implementation complexity.
Several sophisticated variations of Type 3 have emerged for specific business needs:
Extending beyond a single previous value:
ProductDimension {
ProductKey (PK)
ProductID
// Current value
CurrentCategory
// Multiple previous values
PreviousCategory1 // Most recent previous
PreviousCategory2 // Second most recent
PreviousCategory3 // Third most recent
// Change metadata
CategoryChange1Date // When changed to current
CategoryChange2Date // When changed to previous1
CategoryChange3Date // When changed to previous2
}
This approach provides deeper historical context while maintaining the single-row advantage of Type 3.
Using a timestamp-indexed structure for previous values:
ProductDimension {
ProductKey (PK)
ProductID
// Current values
CurrentCategory
// Time-indexed previous values
Category_2023
Category_2022
Category_2021
Category_2020
}
This approach is particularly useful for annual comparisons like fiscal year structures.
Complementing Type 3 with separate history tables:
// Main dimension with Type 3
ProductDimension {
ProductKey (PK)
ProductID
CurrentCategory
PreviousCategory
CategoryChangeDate
}
// Companion history table
ProductCategoryHistory {
ProductKey (FK)
EffectiveDate
CategoryValue
}
This hybrid approach provides both the simplicity of Type 3 for immediate previous value and deeper history in a separate table when needed.
To illustrate a practical Type 3 implementation, consider a sales territory dimension that undergoes periodic reorganization:
DimSalesTerritory {
TerritoryKey (PK)
TerritoryID
// Current structure
CurrentTerritoryName
CurrentRegion
CurrentSalesManager
CurrentSalesTarget
// Previous structure
PreviousTerritoryName
PreviousRegion
PreviousSalesManager
PreviousSalesTarget
// Change tracking
ReorganizationDate
ReorganizationReason
// Regular attributes
GeographicalArea
NumCustomers
NumProspects
LastUpdated
}
This design enables the sales organization to:
- Compare performance before and after territory changes
- Analyze the impact of management changes on sales
- Track how territory targets evolved
- Maintain historical context for fair performance evaluation
To successfully implement Type 3 dimensions, consider these best practices:
Carefully choose which attributes deserve Type 3 treatment:
- Focus on attributes with analytical value in before/after comparison
- Consider change frequency and storage implications
- Prioritize attributes referenced in key business questions
- Document tracking decisions and rationales
Establish clear naming patterns for attribute pairs:
- Current/Previous prefix convention
- Original/Current variation
- Consistent application across attributes
- Clear documentation in data dictionary
Add context to track when and why changes occurred:
- Include change date timestamps
- Consider change reason classifications
- Track change origins (system or user)
- Maintain change sequence information
Establish policies for NULL previous values:
- NULL for never-changed attributes
- NULL vs. empty string for text attributes
- NULL vs. zero for numeric attributes
- Consistent approach in ETL processing
Clearly communicate Type 3 implementation to stakeholders:
- Document attribute pairs in data dictionaries
- Provide example queries for common analyses
- Explain the before/after analytical capabilities
- Be transparent about historical limitations
Several challenges typically arise in Type 3 implementations:
The risk of schema expansion with many Type 3 attributes:
Solution:
- Be highly selective about Type 3 attributes
- Consider alternative approaches for numerous attributes
- Implement Type 3 only for key analytical dimensions
- Use metadata repository to track attribute pairs
Managing scenarios where values change multiple times between ETL runs:
Solution:
- Establish “most significant change” business rules
- Consider frequency-based filtering
- Implement change data capture when possible
- Document limitations in handling interim changes
Business requirements for more than one previous state:
Solution:
- Implement advanced Type 3 variations with multiple previous values
- Consider hybrid approaches with companion history tables
- Evaluate whether Type 2 would be more appropriate
- Clearly document historical depth limitations
Managing unwieldy queries with numerous current/previous pairs:
Solution:
- Create views to simplify common query patterns
- Develop standard query templates
- Consider dynamic SQL for flexible analysis
- Implement semantic layers in BI tools
The Type 3 concept extends beyond traditional data warehousing:
In Data Vault modeling, Type 3 concepts appear in:
- Point-in-Time Satellites with previous value tracking
- Reference Satellites with current and previous classifications
- Link Satellite transition tracking
- Business Vault integrated views with before/after context
Type 3 principles in data lake environments:
- Delta format change tracking
- Hudi time travel with current/previous projections
- Iceberg schema evolution with attribute tracking
- Bronze-to-Silver layer transformation with paired attributes
Implementing Type 3 in cloud platforms:
- Snowflake Time Travel with current/previous views
- BigQuery efficient wide table optimization
- Redshift sort key optimization for paired attributes
- Synapse Polymorphic Table Functions for flexible querying
SCD Type 3 exemplifies the art of balancing competing concerns in data warehouse design. By selectively preserving previous values in dedicated columns, this approach creates dimensions that efficiently support before-and-after analysis without the complexity of full historical versioning.
The “add new attributes” method represents a thoughtful compromise that delivers significant analytical value—enabling direct comparison between current and previous states—while maintaining the query simplicity and performance advantages of single-row-per-entity dimensions. For many business scenarios, particularly those involving planned transitions or reorganizations, this middle path offers the ideal balance of historical context and implementation practicality.
For data engineers and architects designing data warehouses, SCD Type 3 should be viewed not as a lesser alternative to Type 2, but as a specialized tool for specific analytical requirements. When direct comparison between current and previous states is the primary need, Type 3 often provides the most elegant and efficient solution—transforming what might have been complex historical queries into straightforward attribute comparisons.
While not suitable for every historical tracking scenario, Type 3 dimensions deliver substantial business value when applied strategically to the right attributes and analytical contexts. By understanding both the capabilities and limitations of this approach, data engineers can create dimensional models that precisely match business requirements while maintaining performance and usability.
Keywords: SCD Type 3, Slowly Changing Dimensions, add new attributes, dimensional modeling, data warehouse design, before and after analysis, previous values, current values, data warehousing, Kimball methodology, comparative analysis, ETL processing, attribute pairs, data integration, business intelligence
Hashtags: #SCDType3 #SlowlyChangingDimensions #DataWarehouse #DimensionalModeling #DataEngineering #ETLProcessing #BeforeAfterAnalysis #DataIntegration #KimballMethodology #DataArchitecture #BusinessIntelligence #Analytics #DataStrategy #ComparativeAnalysis #PreviousValues