Spark Streaming
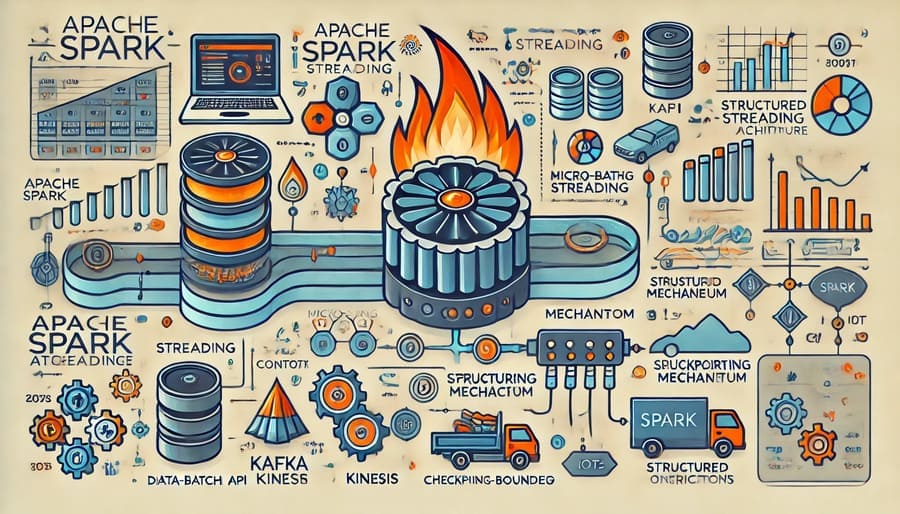
In the rapidly evolving landscape of data processing, the ability to extract value from information as it’s generated has become a critical competitive advantage. Apache Spark Streaming has emerged as a powerful solution that extends the capabilities of the popular Spark framework into the realm of real-time analytics. This comprehensive guide explores how Spark Streaming bridges the gap between batch and real-time processing, enabling organizations to build unified data pipelines that deliver timely insights with the reliability and scalability that modern applications demand.
Traditional data processing followed a batch-oriented approach, where data was collected over time and processed periodically. While effective for many use cases, this approach introduced inherent latency between data generation and insight extraction. As businesses increasingly required faster decision-making, a shift toward real-time processing became necessary.
The journey from batch to real-time processing has seen several evolutionary steps:
- Traditional Batch Processing: Hadoop MapReduce and early data warehousing solutions
- Near Real-Time Processing: Reduced batch sizes with more frequent execution
- Micro-Batch Processing: Processing small batches with sub-minute latency
- True Stream Processing: Event-by-event processing with millisecond latency
Spark Streaming’s innovation lies in its ability to unify batch and streaming paradigms, offering a consistent programming model across different processing requirements.
At its core, Spark Streaming implements a micro-batch processing model that extends the core Spark engine. This approach, often referred to as “discretized streaming,” offers several distinct advantages:
Spark Streaming divides the continuous stream of input data into small, time-bounded batches that are then processed using the core Spark engine. This architecture:
- Leverages the same execution engine for both batch and streaming
- Provides exactly-once processing guarantees
- Enables seamless integration with the broader Spark ecosystem
- Maintains high throughput while offering reasonable latency
The micro-batch architecture stands in contrast to record-by-record processing models employed by frameworks like Apache Flink or Apache Storm. While the latter might offer lower latency for certain use cases, Spark Streaming’s approach brings significant benefits in terms of fault tolerance, consistency, and developer productivity.
Spark Streaming consists of several critical components:
- Discretized Streams (DStreams): The fundamental abstraction representing a continuous sequence of RDDs (Resilient Distributed Datasets)
- Receivers: Components that ingest data from various sources and create DStreams
- StreamingContext: The main entry point for Spark Streaming applications
- Checkpointing: Mechanism for fault tolerance and exactly-once semantics
- Output Operations: Actions that write results to external systems
These components work together to provide a robust foundation for real-time applications.
Spark Streaming has evolved significantly since its introduction. The original DStream API offered powerful capabilities but required developers to work at a relatively low level of abstraction. With the introduction of Structured Streaming in Spark 2.0, the model shifted toward a higher-level, declarative API based on DataFrames and Datasets.
Structured Streaming represents a significant advancement in Spark’s streaming capabilities:
- Unified API: The same DataFrame/Dataset API works for both batch and streaming
- Event-Time Processing: Native support for processing based on when events actually occurred
- Continuous Processing Mode: Option for lower-latency processing (in milliseconds)
- Stateful Operations: Simplified approach to maintaining state across batches
- Watermarking: Handling late-arriving data gracefully
This evolution has made Spark Streaming more accessible to developers while addressing many of the limitations of the original DStream approach.
Spark Streaming can ingest data from various sources:
- Kafka: The most popular message queue for Spark Streaming applications
- Kinesis: Amazon’s managed streaming data service
- HDFS/S3: File-based sources for batch or micro-batch ingestion
- TCP Sockets: Simple network connections for testing
- Custom Receivers: Extensible API for specialized sources
The rich ecosystem of connectors enables integration with virtually any data source.
Once data is ingested, Spark Streaming offers a comprehensive set of transformations:
- Map/FlatMap: Convert each record to one or more output records
- Filter: Remove records that don’t meet specified criteria
- Reduce/Aggregate: Combine records by key using associative operations
- Window: Group records within sliding or tumbling time windows
- Join: Combine records from multiple streams based on keys
- Transform: Apply arbitrary Spark operations to each micro-batch RDD
These operations can be composed to build complex processing pipelines.
After processing, results can be written to various destinations:
- Databases: JDBC connections to relational databases
- NoSQL Stores: MongoDB, Cassandra, HBase, etc.
- Message Queues: Kafka, Kinesis, RabbitMQ
- File Systems: HDFS, S3, and other distributed storage
- Custom Outputs: Specialized connectors for specific systems
End-to-end exactly-once semantics can be achieved with supporting output systems.
import org.apache.spark._
import org.apache.spark.streaming._
// Create a StreamingContext with 2-second batch interval
val conf = new SparkConf().setAppName("WordCountStreaming").setMaster("local[*]")
val ssc = new StreamingContext(conf, Seconds(2))
// Create a DStream from TCP source
val lines = ssc.socketTextStream("localhost", 9999)
// Split lines into words
val words = lines.flatMap(_.split(" "))
// Count words in each batch
val pairs = words.map(word => (word, 1))
val wordCounts = pairs.reduceByKey(_ + _)
// Print the first 10 results
wordCounts.print()
// Start the computation
ssc.start()
ssc.awaitTermination()
import org.apache.spark.sql.functions._
import org.apache.spark.sql.SparkSession
val spark = SparkSession
.builder
.appName("StructuredStreamingExample")
.getOrCreate()
import spark.implicits._
// Create streaming DataFrame from Kafka source
val kafkaStream = spark
.readStream
.format("kafka")
.option("kafka.bootstrap.servers", "localhost:9092")
.option("subscribe", "input-topic")
.load()
// Parse JSON from Kafka messages
val jsonStream = kafkaStream
.selectExpr("CAST(value AS STRING) as json")
.select(from_json($"json", schema).as("data"))
.select("data.*")
// Process data with event-time windows
val windowedCounts = jsonStream
.withWatermark("timestamp", "10 minutes")
.groupBy(
window($"timestamp", "5 minutes", "1 minute"),
$"deviceId")
.count()
// Output to console (for demonstration)
val query = windowedCounts
.writeStream
.outputMode("complete")
.format("console")
.start()
query.awaitTermination()
from pyspark.sql import SparkSession
from pyspark.sql.functions import *
from pyspark.sql.types import *
# Create Spark Session
spark = SparkSession \
.builder \
.appName("PythonStructuredStreamingExample") \
.getOrCreate()
# Define schema for JSON data
schema = StructType([
StructField("device_id", StringType(), True),
StructField("temperature", DoubleType(), True),
StructField("humidity", DoubleType(), True),
StructField("timestamp", TimestampType(), True)
])
# Read from Kafka
kafka_stream = spark \
.readStream \
.format("kafka") \
.option("kafka.bootstrap.servers", "localhost:9092") \
.option("subscribe", "sensor-data") \
.load()
# Parse JSON
json_stream = kafka_stream \
.selectExpr("CAST(value AS STRING) as json") \
.select(from_json(col("json"), schema).alias("data")) \
.select("data.*")
# Calculate average temperature by device in 5-minute windows
window_avg = json_stream \
.withWatermark("timestamp", "10 minutes") \
.groupBy(
window(col("timestamp"), "5 minutes"),
col("device_id")
) \
.agg(
avg("temperature").alias("avg_temperature"),
avg("humidity").alias("avg_humidity")
)
# Write results to console
query = window_avg \
.writeStream \
.outputMode("complete") \
.format("console") \
.option("truncate", "false") \
.start()
query.awaitTermination()
Banks and financial institutions leverage Spark Streaming for:
- Fraud Detection: Identifying suspicious transactions in real-time
- Risk Analysis: Continuously updating exposure calculations
- Algorithmic Trading: Processing market data feeds with low latency
- Compliance Monitoring: Ensuring regulatory requirements are met
The exactly-once processing guarantees are particularly valuable in financial applications where accuracy is paramount.
Retailers use Spark Streaming to enhance customer experiences:
- Real-Time Recommendations: Updating suggestions based on recent behavior
- Inventory Management: Tracking stock levels across channels
- Dynamic Pricing: Adjusting prices based on demand and competition
- Click-Stream Analysis: Understanding customer journey in real-time
These applications enable retailers to personalize experiences and optimize operations dynamically.
Telecom providers implement Spark Streaming for network optimization:
- Network Monitoring: Detecting anomalies and service degradation
- Call Detail Record (CDR) Processing: Analyzing call patterns
- Predictive Maintenance: Identifying potential equipment failures
- Location-Based Services: Delivering contextual information to customers
The ability to process massive volumes of network data helps maintain service quality while reducing costs.
Industrial organizations deploy Spark Streaming for operational technology integration:
- Sensor Data Processing: Analyzing telemetry from thousands of devices
- Predictive Maintenance: Identifying potential equipment failures
- Quality Control: Monitoring production processes in real-time
- Supply Chain Optimization: Tracking materials and finished goods
These applications bridge the gap between operational technology (OT) and information technology (IT).
Effective Spark Streaming deployments require careful resource planning:
- Memory Allocation: Ensuring sufficient heap space for state maintenance
- CPU Cores: Providing adequate processing power for the workload
- Network Bandwidth: Supporting the flow of data between nodes
- Disk I/O: Accommodating checkpoint and shuffle operations
Proper sizing prevents resource contention and ensures consistent performance.
Spark Streaming applications can be deployed in various environments:
- On-Premises Clusters: Traditional Hadoop or standalone Spark clusters
- Cloud Platforms: AWS EMR, Azure HDInsight, Google Dataproc
- Kubernetes: Container-based deployment with dynamic scaling
- Managed Services: Databricks, Cloudera Data Platform, etc.
The choice of deployment model depends on organizational requirements for cost, control, and scalability.
Operational excellence requires comprehensive monitoring:
- Spark UI: Built-in web interface for job monitoring
- Metrics Systems: Integration with Prometheus, Grafana, etc.
- Log Aggregation: Centralized logging with ELK stack or similar
- Alerting: Proactive notification of issues or anomalies
These tools help maintain reliable operation and quickly resolve issues when they occur.
The choice of batch interval significantly impacts both latency and throughput:
- Smaller Intervals: Lower latency but higher scheduling overhead
- Larger Intervals: Higher throughput but increased latency
- Adaptive Intervals: Dynamically adjusting based on processing time
Finding the optimal balance requires understanding application requirements and constraints.
When source data rates exceed processing capacity, backpressure mechanisms prevent system overload:
- Rate Limiting: Dynamically adjusting ingestion rates
- Resource Scaling: Adding processors to handle increased load
- Data Sampling: Processing representative subsets when necessary
These approaches ensure system stability under varying data volumes.
For stateful operations, optimizing state storage and retrieval is critical:
- Minimal State: Storing only essential information
- State Cleanup: Removing outdated or unnecessary state
- Efficient Serialization: Using compact formats like Kryo
- Memory Tuning: Configuring executor memory appropriately
These optimizations prevent memory-related failures in long-running applications.
Comparing two popular stream processing frameworks:
- Processing Model: Micro-batch (Spark) vs. true streaming (Flink)
- Latency: Milliseconds to seconds (Spark) vs. milliseconds (Flink)
- Ecosystem Integration: Broader Spark ecosystem vs. focused streaming capabilities
- Maturity: Established, widely deployed (Spark) vs. growing adoption (Flink)
The choice between these frameworks depends on specific latency requirements and ecosystem considerations.
Different approaches to stream processing:
- Scope: Distributed cluster (Spark) vs. client library (Kafka Streams)
- Integration: Multiple sources/sinks (Spark) vs. Kafka-centric (Kafka Streams)
- Scalability: Cluster-based (Spark) vs. consumer group model (Kafka Streams)
- Complexity: Full-featured framework (Spark) vs. lightweight library (Kafka Streams)
Kafka Streams excels for Kafka-centric processing, while Spark Streaming offers broader capabilities.
Comparing established streaming frameworks:
- Reliability Model: Exactly-once (Spark) vs. at-least-once (Storm)
- Processing Paradigm: Micro-batch (Spark) vs. tuple-by-tuple (Storm)
- Stateful Processing: Integrated (Spark) vs. add-on (Storm Trident)
- Ecosystem: Comprehensive analytics (Spark) vs. focused streaming (Storm)
Spark’s unified analytics platform offers advantages for organizations already using Spark.
Despite its strengths, Spark Streaming presents several challenges:
- Latency Constraints: Micro-batch model imposes minimum latency
- State Management Complexity: Stateful operations require careful design
- Resource Consumption: Memory-intensive for large state or high-volume streams
- Operational Overhead: Cluster management and monitoring requirements
Understanding these limitations helps set appropriate expectations and design more effective applications.
The Spark Streaming ecosystem continues to evolve with several exciting developments:
- Continuous Processing Improvements: Reducing latency further
- Enhanced SQL Support: More comprehensive streaming SQL capabilities
- Python API Enhancements: Better parity with Scala/Java features
- Kubernetes Integration: Improved container-based deployment
- Machine Learning Integration: Tighter coupling with MLlib for streaming ML
These advancements will further strengthen Spark Streaming’s position in the real-time processing landscape.
Setting up a local environment for Spark Streaming development:
- Install Prerequisites:
- Java 8 or later
- Scala 2.12 or later (for Scala development)
- Python 3.6+ (for PySpark)
- Apache Spark 3.x
- Configure Spark:
- Set SPARK_HOME environment variable
- Add Spark bin directory to PATH
- Configure executor memory and cores
- Set Up Test Sources:
- Local Kafka installation
- Simple TCP servers for testing
- Sample data generators
A well-configured development environment accelerates the learning process.
Valuable resources for mastering Spark Streaming:
- Official Documentation: Comprehensive guides and examples
- Books: “Learning Spark” and “Spark: The Definitive Guide”
- Online Courses: Coursera, Udemy, and vendor-specific training
- Community Forums: Stack Overflow and Spark user mailing lists
- GitHub Examples: Sample applications demonstrating best practices
Continuous learning is essential in the rapidly evolving streaming landscape.
Apache Spark Streaming has established itself as a powerful solution for real-time data processing, offering a unique combination of high throughput, reasonable latency, and seamless integration with the broader Spark ecosystem. The evolution from DStreams to Structured Streaming has made the framework more accessible while addressing many of the challenges inherent in distributed stream processing.
For organizations already investing in Spark for batch processing, Spark Streaming provides a natural extension that enables unified data pipelines with consistent programming models. The ability to leverage the same code and infrastructure for both historical and real-time analysis creates significant operational efficiencies and accelerates development.
As data volumes continue to grow and the demand for real-time insights increases, frameworks like Spark Streaming will play an increasingly important role in modern data architectures. By combining the reliability and scalability of Spark with streaming capabilities, organizations can build robust, real-time applications that deliver immediate value from their data assets.
Whether you’re building fraud detection systems, real-time recommendations, IoT analytics, or network monitoring solutions, Spark Streaming offers a compelling combination of developer productivity, operational robustness, and analytical power that makes it a cornerstone of the modern real-time data stack.
#SparkStreaming #ApacheSpark #RealTimeProcessing #BigData #StreamProcessing #DataEngineering #MicroBatch #StructuredStreaming #DistributedSystems #DataPipelines #ETL #AnalyticsEngineering #DataScience #IoTAnalytics #CloudComputing