CircleCI: CI/CD Platform for DevOps
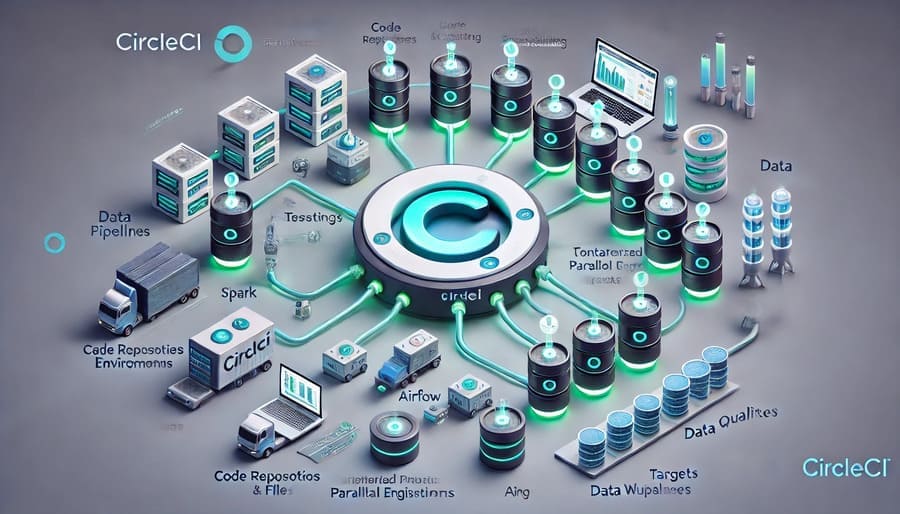
In the fast-paced world of modern software development, continuous integration and continuous delivery (CI/CD) have become essential practices for teams aiming to deliver high-quality code efficiently. Among the leading solutions in this space, CircleCI has established itself as a powerful and flexible CI/CD platform that caters to the needs of DevOps teams across industries. By automating the build, test, and deployment processes, CircleCI enables organizations to ship better code faster while maintaining reliability.
Founded in 2011, CircleCI was born during the early days of the DevOps movement when organizations were beginning to recognize the need for more streamlined software delivery processes. The platform was designed to address a fundamental challenge: how to automate the repetitive tasks of building, testing, and deploying code so that developers could focus on creating value through new features.
Over the years, CircleCI has evolved from a simple CI tool into a comprehensive CI/CD platform that supports complex workflows across diverse technology stacks. Today, it serves thousands of organizations, from startups to enterprises, processing over a million builds daily.
At its heart, CircleCI employs a container-based architecture that provides isolated environments for running builds and tests. This approach offers several advantages:
- Consistency: Each build runs in a clean environment, eliminating “works on my machine” problems
- Parallelism: Tests can be distributed across multiple containers to reduce build times
- Flexibility: Support for custom Docker images allows teams to match their production environments
CircleCI pipelines are defined in a YAML configuration file (.circleci/config.yml
) that specifies the steps, resources, and conditions for your CI/CD process. Here’s a simplified example:
version: 2.1
orbs:
python: circleci/python@1.5
jobs:
build-and-test:
docker:
- image: cimg/python:3.9
steps:
- checkout
- python/install-packages:
pkg-manager: pip
packages:
- pytest
- run:
name: Run tests
command: pytest
workflows:
main:
jobs:
- build-and-test
This configuration demonstrates several key CircleCI concepts:
- Orbs: Reusable packages of configuration that simplify common tasks
- Jobs: Collections of steps that run commands in a specified execution environment
- Workflows: Collections of jobs and their run order, potentially with dependencies
CircleCI’s orbs system represents one of its most innovative features. Orbs are shareable packages of configuration elements that encapsulate common patterns and integrations:
orbs:
aws-cli: circleci/aws-cli@3.1
slack: circleci/slack@4.9
jobs:
deploy:
executor: aws-cli/default
steps:
- aws-cli/setup
- run: aws s3 sync ./build s3://my-bucket
- slack/notify:
event: pass
template: success_tagged_deploy_1
This approach dramatically reduces configuration complexity while enabling teams to leverage community-built best practices.
For data-intensive applications or extensive test suites, CircleCI offers intelligent test splitting that can significantly reduce build times:
jobs:
test:
parallelism: 4
steps:
- checkout
- run:
command: |
circleci tests glob "tests/**/*_test.py" | circleci tests split --split-by=timings
pytest $(circleci tests glob "tests/**/*_test.py" | circleci tests split --split-by=timings)
The platform automatically learns which tests take the longest and distributes them optimally across containers.
Unlike some CI platforms with fixed resource allocations, CircleCI allows teams to choose from various resource classes to match their computational needs:
jobs:
build-large-dataset:
docker:
- image: cimg/python:3.9
resource_class: large
steps:
- checkout
- run: python process_large_dataset.py
Options range from small (1 CPU, 2GB RAM) to 2xlarge (8 CPU, 16GB RAM) for cloud environments, with even larger options available for self-hosted runners.
CircleCI provides sophisticated caching mechanisms to speed up builds by preserving dependencies between runs:
jobs:
build:
steps:
- checkout
- restore_cache:
keys:
- v1-dependencies-{{ checksum "requirements.txt" }}
- run: pip install -r requirements.txt
- save_cache:
paths:
- ./venv
key: v1-dependencies-{{ checksum "requirements.txt" }}
Additionally, workspaces allow data to be shared between jobs in a workflow:
jobs:
build:
steps:
- persist_to_workspace:
root: .
paths:
- dist
deploy:
steps:
- attach_workspace:
at: .
- run: deploy_command ./dist
For data engineering teams, CircleCI offers particularly valuable capabilities:
Data engineers can validate their ETL processes by running them against test datasets:
jobs:
test-etl:
docker:
- image: cimg/python:3.9
- image: postgres:13
environment:
POSTGRES_PASSWORD: postgres
POSTGRES_USER: postgres
POSTGRES_DB: test_db
steps:
- checkout
- run:
name: Install dependencies
command: pip install -r requirements.txt
- run:
name: Run ETL against test dataset
command: python etl_pipeline.py --dataset=test --target=postgres
- run:
name: Validate output data
command: python validate_data_quality.py
CircleCI enables teams to implement automated data quality checks as gates in their deployment process:
jobs:
verify-data-quality:
steps:
- checkout
- run:
name: Run data quality checks
command: |
python -m great_expectations checkpoint run data_quality_suite
- run:
name: Generate data quality report
command: |
python generate_quality_report.py
mkdir -p /tmp/artifacts
cp quality_report.html /tmp/artifacts/
- store_artifacts:
path: /tmp/artifacts
For recurring data tasks, CircleCI supports scheduled workflows:
workflows:
nightly-data-processing:
triggers:
- schedule:
cron: "0 0 * * *"
filters:
branches:
only: main
jobs:
- process-daily-data
Data engineering often involves processing large volumes of data that may be impractical to handle in cloud-based CI environments. CircleCI addresses this with self-hosted runners:
jobs:
process-big-data:
machine: true
resource_class: my-namespace/big-data-runner
steps:
- checkout
- run:
name: Process large dataset
command: spark-submit --master local[*] process_data.py
These runners can be deployed on-premises near data sources, reducing data transfer times and costs while providing access to specialized hardware like GPUs.
Data engineers often work with sensitive information that requires careful handling. CircleCI provides several security features:
Contexts allow teams to store and access environment variables across projects:
workflows:
data-pipeline:
jobs:
- etl-job:
context:
- data-warehouse-credentials
- api-keys
For enhanced security, contexts can be restricted to specific users or teams through role-based access control:
jobs:
sensitive-data-job:
context: restricted-data-access
CircleCI automatically masks secrets in build logs, preventing accidental exposure:
steps:
- run:
name: Access database
command: mysql -u $DB_USER -p$DB_PASSWORD -h $DB_HOST
Even if the command fails and outputs debugging information, the password value remains hidden in logs.
CircleCI shines in its ability to integrate with the broader data engineering toolkit:
orbs:
snowflake: snowflake-inc/snowflake@1.0
jobs:
load-to-warehouse:
steps:
- snowflake/install
- snowflake/run-query:
query-file: load_transformed_data.sql
jobs:
trigger-airflow:
steps:
- run:
name: Trigger Airflow DAG
command: |
curl -X POST \
https://airflow.example.com/api/v1/dags/etl_pipeline/dagRuns \
-H 'Content-Type: application/json' \
-H 'Authorization: Bearer $AIRFLOW_TOKEN' \
-d '{"conf":{"processing_date":"'"$(date +%Y-%m-%d)"'"}}'
orbs:
datadog: datadog/datadog@1.0
jobs:
monitor-pipeline:
steps:
- datadog/install
- datadog/send-metric:
metric: "pipeline.completion"
value: 1
tags: "pipeline:etl,environment:production"
Based on industry experience, here are some best practices for data engineering teams using CircleCI:
Use CircleCI to validate data at each stage of your pipeline:
- Source data validation
- Transformation validation
- Loading validation
- End-to-end tests
- Use appropriate resource classes for data-intensive jobs
- Implement caching for dependencies and intermediate datasets
- Use parallelism for data processing when possible
Design workflows that respect the natural flow of data:
workflows:
etl-pipeline:
jobs:
- extract
- validate-raw:
requires:
- extract
- transform:
requires:
- validate-raw
- validate-transformed:
requires:
- transform
- load:
requires:
- validate-transformed
- end-to-end-validation:
requires:
- load
For tests requiring databases or other stateful services:
- Use dedicated testing instances
- Implement proper setup and teardown
- Consider database snapshots for complex scenarios
A financial services company processing millions of transactions daily implemented CircleCI to automate their data pipeline validation. Their approach included:
- Tiered testing strategy:
- Unit tests for individual transformations
- Integration tests for data flow between systems
- End-to-end tests with synthetic data
- Environment-specific configurations:
- Development: Full pipeline with small synthetic datasets
- Staging: Full pipeline with anonymized production samples
- Production: Validation-only checks on actual data
- Self-hosted runners for sensitive data processing, keeping customer data within their secure network
The result was a 60% reduction in data quality issues reaching production and a 40% decrease in time-to-deployment for new data models.
Looking ahead, several trends are shaping how CircleCI will continue to serve data engineering teams:
- Increased focus on machine learning pipelines, with specialized tools for model validation and deployment
- Enhanced observability through deeper integration with monitoring tools
- Expanded ecosystem integrations with data engineering-specific tools and platforms
- Improved support for large-scale data processing through optimized runners and resource allocation
CircleCI has evolved into a versatile platform that addresses many of the unique challenges faced by data engineering teams. From testing ETL processes to validating data quality and automating deployments, it provides the tools needed to implement robust CI/CD practices for data pipelines.
By leveraging CircleCI’s features like orbs, intelligent test splitting, comprehensive caching, and self-hosted runners, data engineering teams can build more reliable data systems while accelerating their delivery cycles. As data volumes continue to grow and data engineering practices mature, platforms like CircleCI will play an increasingly important role in ensuring that data pipelines meet the same quality standards that we’ve come to expect from application development.
Whether you’re building data warehouses, implementing real-time analytics, or developing machine learning pipelines, CircleCI offers the flexibility and power to automate your workflow from source to production, helping your team focus on what matters most: delivering valuable insights from your data.
Keywords: CircleCI, CI/CD, continuous integration, continuous delivery, DevOps, data engineering, ETL pipelines, automated testing, workflow automation, container-based CI, orbs, data quality, pipeline automation, test parallelism, self-hosted runners
#CircleCI #CICD #DevOps #DataEngineering #AutomatedTesting #DataPipelines #Automation #ContainerizedCI #TestParallelism #ETLAutomation #DataOps #ContinuousIntegration #ContinuousDelivery #DataQuality #CloudNative