Jenkins: Open-Source Automation Server
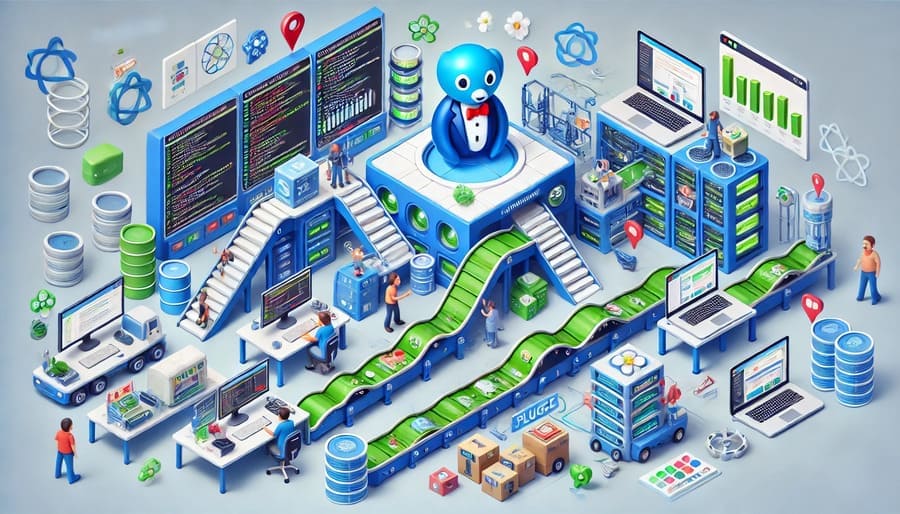
In the ever-evolving landscape of software development and IT operations, automation has become not just a luxury but a necessity. Among the pioneers that revolutionized this space, Jenkins stands tall as one of the most versatile, battle-tested open-source automation servers available today. From its humble beginnings as the “Hudson” project at Sun Microsystems to becoming the backbone of countless organizations’ delivery pipelines worldwide, Jenkins has earned its place as a cornerstone of modern DevOps practices.
Jenkins began its journey in 2004 when Kohsuke Kawaguchi, then a developer at Sun Microsystems, created a continuous integration tool called “Hudson.” Frustrated with broken builds interrupting his work, Kawaguchi developed Hudson to automatically test code changes as they were committed. What started as a personal solution soon gained popularity within Sun and eventually across the wider developer community.
In 2011, following Oracle’s acquisition of Sun Microsystems, a dispute over the project’s governance led Kawaguchi and most contributors to fork the codebase. The fork was named “Jenkins,” while Oracle continued the original project as “Hudson.” Over time, the Jenkins fork flourished with community support, while Hudson gradually faded into obscurity.
Today, Jenkins is maintained by the Jenkins project under the governance of the Continuous Delivery Foundation, ensuring its continued development as a truly community-driven tool.
At its heart, Jenkins provides a framework for automating various parts of the software development lifecycle. Its primary capabilities include:
Jenkins automatically builds and tests code changes as they’re committed to the repository, quickly identifying integration issues:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn -B -DskipTests clean package'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
post {
always {
junit 'target/surefire-reports/*.xml'
}
}
}
}
}
Jenkins orchestrates the deployment of applications to various environments, ensuring consistent release processes:
stage('Deploy to Staging') {
steps {
withCredentials([sshUserPrivateKey(credentialsId: 'staging-server', keyFileVariable: 'KEY')]) {
sh 'scp -i $KEY target/*.jar user@staging-server:/opt/app/'
sh 'ssh -i $KEY user@staging-server "systemctl restart myapp"'
}
}
}
Beyond simple build and deploy tasks, Jenkins can coordinate complex workflows involving multiple systems, approval gates, and conditional logic:
pipeline {
agent any
stages {
stage('Build and Test') { /* ... */ }
stage('Deploy to Staging') { /* ... */ }
stage('Integration Tests') { /* ... */ }
stage('Manual Approval') {
steps {
timeout(time: 24, unit: 'HOURS') {
input message: 'Approve deployment to production?'
}
}
}
stage('Deploy to Production') { /* ... */ }
}
}
Understanding Jenkins’ architecture helps explain its flexibility and widespread adoption:
Jenkins operates on a master-agent architecture:
- Master: The central coordinator that schedules jobs, distributes work, maintains the UI, and stores configurations
- Agents: Worker nodes that execute the actual tasks, allowing for distributed builds across multiple environments
This architecture enables Jenkins to scale horizontally, handling everything from small team projects to enterprise-wide automation needs.
Perhaps Jenkins’ greatest strength is its extensive plugin ecosystem, with over 1,800 community-contributed plugins that extend its functionality:
- Source Control Management: Git, Subversion, Mercurial, etc.
- Build Tools: Maven, Gradle, npm, etc.
- Testing Frameworks: JUnit, Selenium, SonarQube, etc.
- Deployment Platforms: AWS, Azure, Kubernetes, etc.
- Notification Services: Email, Slack, Teams, etc.
This extensibility allows Jenkins to adapt to virtually any technology stack or workflow requirement.
While Jenkins gained popularity in traditional software development, it has proven equally valuable for data engineering workflows. Here’s how data teams leverage Jenkins:
Jenkins excels at orchestrating Extract, Transform, Load (ETL) processes that form the backbone of data warehousing:
pipeline {
agent any
triggers {
cron('0 2 * * *') // Run daily at 2 AM
}
stages {
stage('Extract') {
steps {
sh 'python extract_data.py --source=production --date=$(date +%Y-%m-%d)'
}
}
stage('Transform') {
steps {
sh 'spark-submit transform_data.py --input=raw_data --output=transformed_data'
}
}
stage('Validate') {
steps {
sh 'python validate_data_quality.py --dataset=transformed_data'
}
}
stage('Load') {
steps {
sh 'python load_to_warehouse.py --dataset=transformed_data --target=production'
}
}
}
post {
success {
mail to: 'data-team@example.com',
subject: "ETL Pipeline Completed: ${currentBuild.fullDisplayName}",
body: "The ETL pipeline completed successfully."
}
failure {
mail to: 'data-team@example.com',
subject: "ETL Pipeline Failed: ${currentBuild.fullDisplayName}",
body: "The ETL pipeline failed. Check the logs at: ${BUILD_URL}"
}
}
}
Jenkins’ robust scheduling capabilities make it ideal for coordinating time-sensitive data processing:
- Incremental processing throughout the day
- End-of-day reconciliation jobs
- Monthly reporting and aggregation
- Data synchronization between systems
Jenkins can enforce data quality standards through automated validation:
stage('Data Quality Check') {
steps {
script {
def qualityResults = sh(script: 'python quality_check.py --dataset=customer_data', returnStatus: true)
if (qualityResults != 0) {
error "Data quality checks failed. See attached report for details."
}
}
}
post {
always {
archiveArtifacts artifacts: 'quality_report.html', fingerprint: true
}
}
}
For data science teams, Jenkins automates the ML lifecycle:
pipeline {
agent any
stages {
stage('Prepare Data') { /* ... */ }
stage('Train Model') {
steps {
sh 'python train_model.py --dataset=training_data --model-type=random_forest'
}
post {
success {
archiveArtifacts artifacts: 'models/model.pkl', fingerprint: true
}
}
}
stage('Evaluate Model') {
steps {
sh 'python evaluate_model.py --model=models/model.pkl --test-data=test_data'
}
post {
always {
junit 'model_metrics.xml'
}
}
}
stage('Deploy Model') {
when {
expression {
return currentBuild.resultIsBetterOrEqualTo('SUCCESS') &&
sh(script: 'python check_model_improvement.py', returnStatus: true) == 0
}
}
steps {
sh 'python deploy_model.py --model=models/model.pkl --environment=production'
}
}
}
}
While traditional Jenkins freestyle jobs served their purpose well, the introduction of Jenkins Pipeline marked a significant evolution in how automation is defined and managed:
Jenkins offers two syntax styles for defining pipelines:
Declarative Pipeline provides a more structured syntax with predefined sections:
pipeline {
agent any
stages {
stage('Build') {
steps {
echo 'Building..'
}
}
stage('Test') {
steps {
echo 'Testing..'
}
}
stage('Deploy') {
steps {
echo 'Deploying....'
}
}
}
}
Scripted Pipeline offers more flexibility through Groovy scripting:
node {
try {
stage('Build') {
// Custom build logic
}
stage('Test') {
parallel unitTests: {
// Run unit tests
}, integrationTests: {
// Run integration tests
}
}
if (env.BRANCH_NAME == 'main') {
stage('Deploy') {
// Deploy only for main branch
}
}
} catch (e) {
// Custom error handling
throw e
} finally {
// Cleanup operations
}
}
The “Pipeline as Code” approach stores pipeline definitions in version control alongside application code, offering several advantages:
- Versioning: Pipeline changes are tracked, reviewed, and audited
- Self-documentation: The pipeline itself documents the build and deployment process
- Reusability: Pipeline libraries allow sharing common functionality across projects
- Disaster recovery: Pipelines can be restored along with the application code
As cloud-native technologies gained momentum, the Jenkins ecosystem evolved to embrace them with Jenkins X, a reimagined CI/CD solution designed specifically for Kubernetes environments.
Jenkins X provides:
- Automated CI/CD pipelines for cloud applications
- Environment promotion across development, staging, and production
- Integration with GitHub, GitLab, and other platforms for pull request-based workflows
- Preview environments for reviewing changes before they’re merged
- GitOps-based deployment practices
While traditional Jenkins remains widely used across various infrastructure types, Jenkins X represents the project’s adaptation to modern cloud-native development patterns.
Organizations that have successfully implemented Jenkins at scale follow these key practices:
Use tools like Jenkins Configuration as Code (JCasC) to define your Jenkins configuration declaratively:
jenkins:
systemMessage: "Jenkins configured automatically by Jenkins Configuration as Code"
securityRealm:
ldap:
configurations:
- server: ldap.example.org
rootDN: dc=example,dc=org
userSearchBase: ou=people
authorizationStrategy:
roleBased:
roles:
global:
- name: "admin"
description: "Jenkins administrators"
permissions:
- "Overall/Administer"
assignments:
- "admin"
- name: "developer"
description: "Jenkins developers"
permissions:
- "Overall/Read"
- "Job/Build"
assignments:
- "dev-team"
For mission-critical environments, implement high availability:
- Store Jenkins configuration in external storage (AWS EFS, NFS, etc.)
- Use a database-backed job history instead of the default file-based storage
- Deploy multiple Jenkins masters with load balancing
- Implement automated backup and recovery procedures
Protect your automation infrastructure:
- Implement proper authentication (LDAP, OAuth, etc.) and authorization
- Use credential management for secrets (never hardcode)
- Regularly update Jenkins and plugins to patch vulnerabilities
- Isolate build environments using containerization
- Implement network security controls around Jenkins infrastructure
Keep Jenkins running smoothly:
- Configure appropriate heap size and garbage collection
- Archive and prune old builds regularly
- Use the “Discard old builds” feature to manage workspace growth
- Monitor agent utilization and scale accordingly
- Optimize pipeline scripts for efficiency
While Jenkins remains widely used, the CI/CD landscape has evolved with new competitors:
Feature | Jenkins | GitHub Actions | GitLab CI/CD | CircleCI |
---|---|---|---|---|
Hosting | Self-hosted | Cloud (GitHub) | Self-hosted or Cloud | Cloud |
Configuration | Groovy DSL, UI | YAML | YAML | YAML |
Learning Curve | Steeper | Moderate | Moderate | Moderate |
Extensibility | Very High (plugins) | Growing | Good | Good |
Cloud-Native Support | With Jenkins X | Native | Native | Native |
Cost | Free (infrastructure costs) | Free tier + paid | Free tier + paid | Free tier + paid |
Community Size | Very Large | Growing | Large | Medium |
Jenkins’ main advantages remain its flexibility, extensibility, and the ability to run on-premises—critical for organizations with strict data sovereignty requirements or specialized infrastructure needs.
Despite the emergence of cloud-native CI/CD tools, Jenkins continues to evolve to meet modern development needs:
- Improved cloud integration: Better support for containerized workloads and cloud services
- Enhanced UX: Modernizing the user interface and improving usability
- Performance improvements: Addressing legacy architectural limitations
- Pipeline enhancements: More powerful pipeline capabilities and integration patterns
- Security hardening: Continuing to improve security posture and vulnerability management
The Jenkins project’s commitment to backwards compatibility while evolving for future needs ensures it will remain relevant in the CI/CD landscape for years to come.
Jenkins has earned its place in the automation hall of fame through two decades of continuous evolution, community support, and adaptability to changing technology landscapes. From traditional software development to modern data engineering and cloud-native applications, Jenkins provides a powerful, flexible framework for automating critical workflows.
While newer tools may offer specific advantages for particular use cases, Jenkins’ extensibility, maturity, and vendor-neutral approach continue to make it a compelling choice for organizations seeking a robust automation foundation. Whether you’re building a simple application deployment pipeline or orchestrating complex data engineering workflows across multiple environments, Jenkins offers the tools and ecosystem to bring your automation vision to life.
As the software development and data engineering disciplines continue to evolve, Jenkins will likely remain a key player in the automation landscape—adapting, as it always has, to meet the changing needs of the communities it serves.
Keywords: Jenkins, continuous integration, continuous delivery, CI/CD, automation server, DevOps, pipeline, build automation, deployment automation, open-source, Hudson, data engineering, ETL pipeline, workflow orchestration, Jenkins Pipeline, Jenkins X, Kubernetes
#Jenkins #CICD #DevOps #Automation #ContinuousIntegration #ContinuousDelivery #OpenSource #Pipeline #DataEngineering #ETL #BuildAutomation #JenkinsPipeline #AutomationServer #WorkflowOrchestration #DataOps