Puppet: Configuration Management Tool for Modern Infrastructure
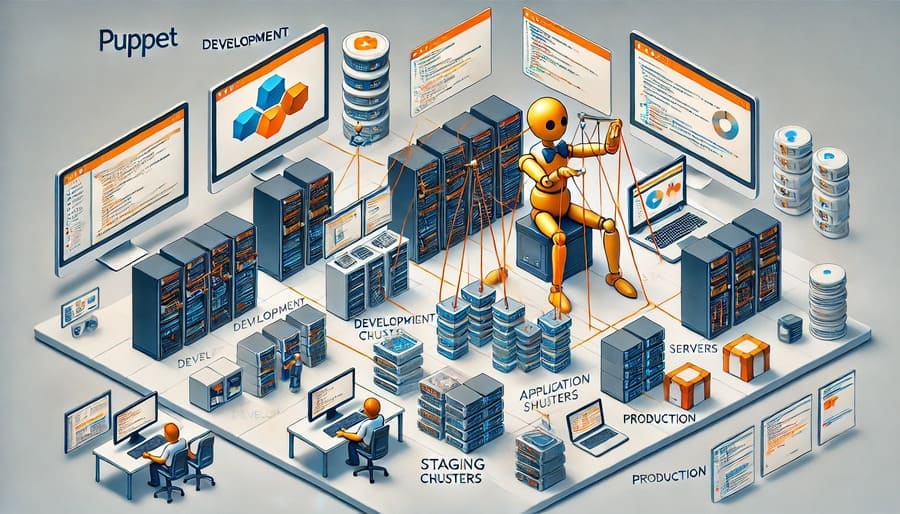
In the ever-evolving landscape of IT infrastructure and DevOps, configuration management tools have become essential for organizations seeking consistency, reliability, and scalability. Among these powerful tools, Puppet has established itself as a pioneering solution that has helped shape the very concept of infrastructure as code. From its humble beginnings to its current enterprise-grade capabilities, Puppet continues to be a cornerstone technology for automating the deployment and management of complex IT environments.
Puppet emerged in 2005 when Luke Kanies, frustrated with the limitations of existing tools, created a new approach to system administration. His vision was to develop a framework that would enable administrators to define infrastructure configurations declaratively rather than through imperative scripts.
This innovative approach allowed systems to be described in terms of their desired end state rather than the specific steps needed to reach that state. The software would then determine how to bring systems into compliance with that definition—a paradigm shift that has since become standard practice in the industry.
Over the years, Puppet has evolved from a relatively simple open-source tool to a comprehensive ecosystem that supports complex enterprise deployments. The Puppet Enterprise offering now provides enhanced features, support, and scalability for organizations managing thousands of nodes across various environments.
At its heart, Puppet operates on a few fundamental concepts that make it powerful and flexible:
Puppet uses a domain-specific language (DSL) that allows administrators to describe the desired system state rather than the steps to achieve it:
package { 'nginx':
ensure => installed,
}
file { '/etc/nginx/nginx.conf':
ensure => file,
content => template('nginx/nginx.conf.erb'),
require => Package['nginx'],
}
service { 'nginx':
ensure => running,
enable => true,
subscribe => File['/etc/nginx/nginx.conf'],
}
This example demonstrates how Puppet ensures Nginx is installed, properly configured, and running—describing what should be true rather than how to make it true.
Puppet typically operates in a client-server model:
- Puppet Server (formerly “Puppet Master”): The central component that compiles configurations into catalogs and serves them to agents
- Puppet Agents: Client software running on managed nodes that applies the configurations
- PuppetDB: A database that stores the data Puppet collects, supporting queries and reports
This architecture enables centralized management of configurations across an entire infrastructure, from a handful of servers to tens of thousands.
One of Puppet’s most powerful features is idempotency—the ability to apply configurations repeatedly without causing unintended side effects. No matter how many times a Puppet run executes, the system will converge on the same desired state, making configuration management predictable and reliable.
Puppet creates customized configurations for each system through two key mechanisms:
- Facts: System-specific information gathered by Puppet agents (such as operating system, IP addresses, hardware details)
- Catalogs: Compiled configurations that define exactly what changes should be applied to a specific node
Together, these allow for targeted configurations that adapt to the specific characteristics of each managed system.
For data engineering teams, Puppet offers particularly valuable capabilities for managing complex, distributed data infrastructure:
Puppet can ensure database servers are consistently configured across environments:
class profile::database::postgresql (
String $version = '13',
Integer $max_connections = 100,
String $data_directory = '/var/lib/postgresql/data',
) {
class { 'postgresql::server':
version => $version,
manage_package_repo => true,
ip_mask_allow_all_users => '0.0.0.0/0',
postgres_password => Sensitive($postgresql_password),
config_entries => {
'max_connections' => $max_connections,
'shared_buffers' => '1GB',
'work_mem' => '64MB',
'maintenance_work_mem' => '128MB',
'effective_cache_size' => '4GB',
},
}
postgresql::server::db { 'analytics':
user => 'analytics_user',
password => postgresql_password('analytics_user', $analytics_db_password),
}
}
For technologies like Hadoop, Spark, or Kafka, Puppet can coordinate the configuration of distributed clusters:
class profile::bigdata::spark_worker (
String $spark_version = '3.1.2',
String $hadoop_version = '3.2',
Array[String] $master_hosts,
String $worker_memory = '4g',
Integer $worker_cores = 2,
) {
include profile::java
package { 'spark':
ensure => $spark_version,
}
file { '/etc/spark/conf/spark-env.sh':
ensure => file,
content => template('profile/bigdata/spark-env.sh.erb'),
require => Package['spark'],
}
file { '/etc/spark/conf/spark-defaults.conf':
ensure => file,
content => template('profile/bigdata/spark-defaults.conf.erb'),
require => Package['spark'],
}
service { 'spark-worker':
ensure => running,
enable => true,
require => [
File['/etc/spark/conf/spark-env.sh'],
File['/etc/spark/conf/spark-defaults.conf'],
],
}
}
Puppet can manage the deployment and configuration of job scheduling systems like Apache Airflow:
class profile::etl::airflow (
String $airflow_version = '2.2.3',
String $db_connection = 'postgresql+psycopg2://airflow:airflow@localhost/airflow',
String $executor = 'CeleryExecutor',
Integer $parallelism = 32,
) {
include profile::python
python::pip { 'apache-airflow':
ensure => $airflow_version,
virtualenv => '/opt/airflow/venv',
}
file { '/etc/airflow/airflow.cfg':
ensure => file,
content => template('profile/etl/airflow.cfg.erb'),
require => Python::Pip['apache-airflow'],
}
service { 'airflow-webserver':
ensure => running,
enable => true,
require => File['/etc/airflow/airflow.cfg'],
}
service { 'airflow-scheduler':
ensure => running,
enable => true,
require => File['/etc/airflow/airflow.cfg'],
}
}
Puppet can manage complex data warehouse deployments, ensuring consistent configuration:
class profile::datawarehouse::snowflake_client (
String $version = '2.0.0',
String $account_name,
String $region = 'us-west-2',
Hash $connection_profiles = {},
) {
package { 'snowflake-cli':
ensure => $version,
}
$connection_profiles.each |String $profile_name, Hash $profile_config| {
file { "/etc/snowflake/profiles/${profile_name}.json":
ensure => file,
content => inline_template('<%= @profile_config.to_json %>'),
mode => '0600',
require => Package['snowflake-cli'],
}
}
}
As organizations scale their Puppet deployments, several advanced features become essential:
Hiera separates data from code, allowing for environment-specific configurations without duplicating Puppet manifests:
# common.yaml
---
profile::database::postgresql::max_connections: 100
profile::database::postgresql::version: '13'
# environment/production.yaml
---
profile::database::postgresql::max_connections: 500
With this approach, you can override specific parameters for different environments, making configuration more maintainable.
For large-scale deployments, the Roles and Profiles pattern provides a structured way to organize Puppet code:
- Profiles: Technology-specific configurations (e.g., a PostgreSQL server)
- Roles: Business-specific collections of profiles (e.g., a data processing node)
# A profile for Apache Spark
class profile::spark { ... }
# A profile for Hadoop HDFS
class profile::hdfs { ... }
# A role that combines them for a data processing node
class role::data_processing_node {
include profile::spark
include profile::hdfs
}
This pattern creates a clear separation between implementation details and business logic, making the infrastructure more maintainable.
Enterprise Puppet deployments typically use a control repository to manage the Puppet codebase:
control-repo/
├── Puppetfile # External module dependencies
├── environment.conf # Environment-specific settings
├── hiera.yaml # Hiera configuration
├── data/ # Hiera data files
├── manifests/
│ └── site.pp # Node classifications
└── site/ # Site-specific modules
├── profile/ # Profile modules
└── role/ # Role modules
Combined with Puppet’s Code Manager or r10k, this approach enables Git-based workflow and continuous delivery of configuration changes.
For dynamic infrastructure, Puppet provides mechanisms to determine node configurations based on external systems:
- Trusted Facts: Secure, verified information about nodes (e.g., certificates)
- External Node Classifiers (ENC): External systems that determine which classes a node should include
These features are particularly valuable in cloud environments where servers may be created and destroyed frequently.
Based on industry experience, here are some best practices for using Puppet effectively in data engineering contexts:
Implement testing for your Puppet code to ensure it works as expected:
# A RSpec test for a PostgreSQL profile
describe 'profile::database::postgresql' do
it 'should compile' do
is_expected.to compile.with_all_deps
end
it 'should contain the PostgreSQL server class' do
is_expected.to contain_class('postgresql::server')
end
it 'should create the analytics database' do
is_expected.to contain_postgresql__server__db('analytics')
end
end
Tools like rspec-puppet, puppet-lint, and kitchen-puppet can help catch issues before they reach production.
Treat your Puppet code with the same rigor as application code:
- Use version control for all Puppet code
- Implement continuous integration to validate changes
- Use code reviews before merging changes
- Automatically test changes in staging environments before production
This approach reduces the risk of configuration drift and improves the reliability of deployments.
While consistency is a key benefit of configuration management, different environments often have legitimate variations:
class profile::spark (
Enum['development', 'staging', 'production'] $environment = 'production',
) {
if $environment == 'development' {
$executor_memory = '1g'
$executor_instances = 2
} elsif $environment == 'staging' {
$executor_memory = '4g'
$executor_instances = 4
} else {
$executor_memory = '8g'
$executor_instances = 8
}
# Configuration using environment-specific values
}
Use Hiera and conditional logic judiciously to accommodate these differences without sacrificing maintainability.
Good documentation is essential for maintaining complex infrastructure:
# @summary Configures a Kafka broker in a cluster
#
# @param broker_id The unique ID for this broker in the cluster
# @param zookeeper_servers Array of ZooKeeper servers to connect to
# @param log_dirs Directories where log segments are stored
# @param heap_size Java heap size for the Kafka broker
#
# @example
# include profile::messaging::kafka_broker
class profile::messaging::kafka_broker (
Integer $broker_id,
Array[String] $zookeeper_servers,
Array[String] $log_dirs = ['/var/lib/kafka/logs'],
String $heap_size = '6G',
) {
# Implementation
}
Use comments, examples, and parameter documentation to make your code self-explanatory.
Integrate Puppet with your monitoring systems to track configuration status:
- Export metrics from PuppetDB to monitoring systems
- Set up alerts for failed Puppet runs or unexpected configuration changes
- Generate regular compliance reports for auditing purposes
This visibility helps identify and address issues proactively.
While Puppet has been a pioneer in configuration management, several alternatives have emerged:
Feature | Puppet | Chef | Ansible | SaltStack |
---|---|---|---|---|
Language Type | Declarative DSL | Procedural (Ruby) | Procedural (YAML) | Declarative & Procedural |
Agent Requirement | Yes (typically) | Yes | No (agentless) | Optional |
Learning Curve | Moderate | Steeper | Gentler | Moderate |
Maturity | Very Mature | Very Mature | Mature | Mature |
Cloud Integration | Strong | Strong | Strong | Strong |
Community Size | Large | Large | Very Large | Moderate |
The choice between these tools often depends on specific organizational requirements, existing skills, and infrastructure needs. Puppet’s strengths include its mature ecosystem, strong support for complex infrastructure, and robust enterprise features.
As infrastructure continues to evolve toward cloud-native architectures, Puppet is adapting to remain relevant:
Puppet Bolt provides task-based automation that complements Puppet’s declarative model:
bolt task run package name=nginx action=install --targets webservers
This approach bridges the gap between configuration management and ad-hoc task execution, addressing some of the flexibility concerns that have driven adoption of tools like Ansible.
Puppet now offers solutions for managing containerized environments:
class profile::kubernetes::deployment (
String $image,
String $version,
Integer $replicas = 3,
) {
kubernetes::resource { 'my-deployment':
resource_type => 'deployment',
content => {
'apiVersion' => 'apps/v1',
'kind' => 'Deployment',
'metadata' => {
'name' => 'my-app',
},
'spec' => {
'replicas' => $replicas,
'selector' => {
'matchLabels' => {
'app' => 'my-app',
},
},
'template' => {
'metadata' => {
'labels' => {
'app' => 'my-app',
},
},
'spec' => {
'containers' => [
{
'name' => 'my-app',
'image' => "${image}:${version}",
},
],
},
},
},
},
}
}
This capability allows organizations to use Puppet for both traditional infrastructure and containerized environments.
Newer Puppet tools focus on discovering and analyzing infrastructure, then continuously delivering configuration changes:
- Puppet Discovery: Helps identify unmanaged resources
- Continuous Delivery for Puppet Enterprise: Automates the testing and deployment of Puppet code changes
These tools help organizations move toward more dynamic, cloud-like operations even with traditional infrastructure.
Puppet remains a powerful tool for managing complex IT infrastructure, particularly in environments with diverse technologies and stringent compliance requirements. For data engineering teams, Puppet offers the ability to consistently deploy and manage the various components of data processing platforms—from databases and message queues to processing frameworks and visualization tools.
While newer tools have emerged with different approaches to configuration management, Puppet’s mature ecosystem, robust enterprise features, and ongoing evolution ensure it will continue to play a significant role in infrastructure automation.
Organizations that invest in Puppet gain not just a tool, but a comprehensive approach to infrastructure as code—bringing software engineering practices to infrastructure management and enabling more reliable, scalable, and maintainable data platforms.
As data engineering continues to grow in complexity and importance, tools like Puppet that enforce consistency, enable automation, and provide visibility will remain essential components of the modern data engineering toolkit.
Keywords: Puppet, configuration management, infrastructure as code, automation, DevOps, data engineering, system administration, PostgreSQL, Hadoop, Kafka, Spark, deployment automation, idempotency, continuous delivery, Puppet Enterprise, Bolt, server management
#Puppet #ConfigurationManagement #InfrastructureAsCode #DevOps #DataEngineering #Automation #IaC #ServerManagement #ITAutomation #PuppetEnterprise #SystemAdministration #ContinuousDelivery #DataOps #CloudAutomation #Idempotency