Programming Languages
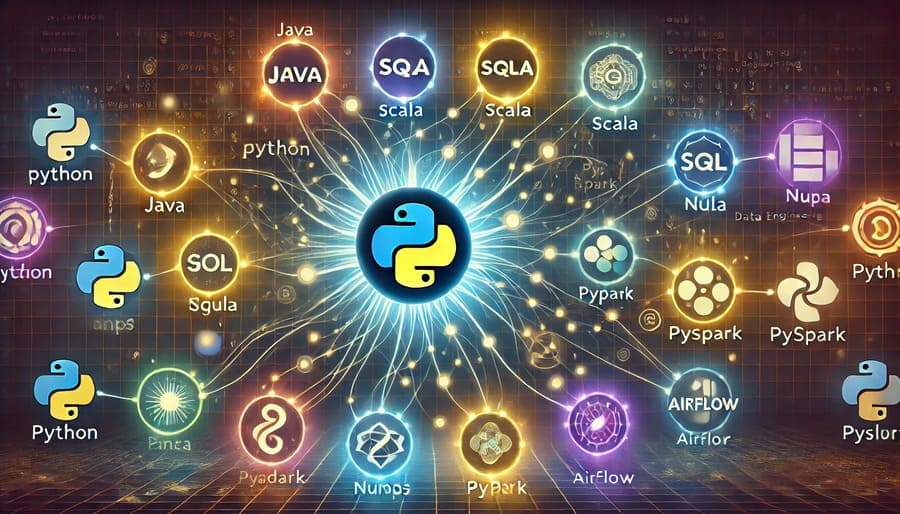
- Python: General-purpose language popular in data engineering
- Java: General-purpose language used in many big data tools
- Scala: JVM language with functional programming features
- SQL: Language for managing and querying relational databases
- R: Language for statistical computing and graphics
- Go: Efficient and reliable language for distributed systems
- Julia: High-level, high-performance language for numerical analysis
In today’s data-driven world, the toolkit of a successful data engineer continues to evolve. From processing vast datasets to building robust pipelines, selecting the right programming languages and libraries can make all the difference. This comprehensive guide explores the most powerful tools that form the backbone of modern data engineering.
Python has emerged as the undisputed favorite in data engineering for good reason. Its readable syntax, extensive library ecosystem, and versatility make it ideal for everything from data manipulation to machine learning integration. Python excels in rapid prototyping while still being robust enough for production environments.
# Simple example of Python data processing
import pandas as pd
def transform_data(input_file, output_file):
# Read data
df = pd.read_csv(input_file)
# Transform data
df['processed'] = df['raw_value'] * 2
# Write results
df.to_csv(output_file, index=False)
Despite being older than many alternatives, Java remains crucial in data engineering, particularly for organizations prioritizing stability and performance. Its strongly-typed nature and mature ecosystem make it the foundation for numerous big data frameworks including Hadoop, Kafka, and Spark. Java’s “write once, run anywhere” capability ensures consistent performance across different environments.
Scala combines object-oriented and functional programming paradigms, making it particularly well-suited for distributed computing. Running on the Java Virtual Machine (JVM), Scala offers better concurrency handling than Java while maintaining compatibility with existing Java libraries. It’s the native language of Spark and provides more concise syntax for complex data transformations.
SQL (Structured Query Language) remains the universal language for interacting with relational databases. Every data engineer must master SQL fundamentals:
-- Example of a SQL data transformation
SELECT
customer_id,
SUM(purchase_amount) as total_purchases,
COUNT(*) as purchase_count,
AVG(purchase_amount) as average_purchase
FROM transactions
WHERE transaction_date > '2023-01-01'
GROUP BY customer_id
HAVING COUNT(*) > 5
ORDER BY total_purchases DESC;
Modern data engineering has expanded SQL’s reach through tools like Spark SQL, Presto, and BigQuery, which apply SQL’s familiar syntax to non-relational data sources.
While Python has gained popularity for general data tasks, R continues to excel specifically in statistical analysis and visualization. R’s specialized packages for statistical modeling provide capabilities that are sometimes more advanced than Python equivalents, making it valuable for data engineers working closely with data scientists.
Go (or Golang) has gained traction in data engineering for its efficiency, simplicity, and excellent support for concurrency. Designed by Google to handle large-scale systems, Go produces compiled, statically-typed code that runs quickly and requires minimal resources. It’s increasingly used for building data pipelines, microservices, and ETL processes.
Julia addresses the “two-language problem” by combining Python’s ease of use with C’s performance. It offers a sweet spot for data engineers working with computationally intensive numerical operations, delivering performance comparable to statically compiled languages while maintaining dynamic language convenience.
Pandas revolutionized data manipulation in Python with its DataFrame structure, making it the standard starting point for most data engineering tasks. It excels at cleaning, transforming, and analyzing structured data through intuitive operations:
import pandas as pd
# Load data
df = pd.read_csv('transactions.csv')
# Clean data
df.dropna(subset=['customer_id'], inplace=True)
# Transform data
df['transaction_date'] = pd.to_datetime(df['transaction_date'])
df['transaction_month'] = df['transaction_date'].dt.month
# Aggregate data
monthly_sales = df.groupby('transaction_month')['amount'].sum()
NumPy serves as the foundation for numerical computing in Python, providing efficient array operations essential for data processing. Its vectorized operations significantly outperform standard Python loops when working with large datasets.
dbt has transformed how data teams handle transformations within data warehouses, bringing software engineering practices to SQL:
-- Example dbt model
{{ config(materialized='table') }}
SELECT
user_id,
COUNT(*) as login_count,
MIN(created_at) as first_login,
MAX(created_at) as most_recent_login
FROM {{ ref('stg_logins') }}
GROUP BY user_id
By organizing transformations as models with documentation, testing, and version control, dbt helps maintain data quality and lineage.
PySpark brings Apache Spark’s distributed computing power to Python, enabling data engineers to process massive datasets across clusters:
from pyspark.sql import SparkSession
from pyspark.sql.functions import col, sum
# Initialize Spark
spark = SparkSession.builder.appName("Sales Analysis").getOrCreate()
# Read data
sales = spark.read.parquet("s3://data/sales/")
# Transform and aggregate
result = sales.filter(col("date") > "2023-01-01") \
.groupBy("product_category") \
.agg(sum("amount").alias("total_sales"))
# Write results
result.write.mode("overwrite").parquet("s3://data/aggregated/sales/")
Dask scales Python libraries like Pandas and NumPy to multi-core machines and distributed clusters without requiring a completely new API. This makes it ideal for scaling existing workflows:
import dask.dataframe as dd
# Create a Dask DataFrame from many CSV files
df = dd.read_csv('s3://data/logs/*.csv')
# Perform operations like with pandas
result = df.groupby('user_id').agg({'duration': 'mean'})
# Compute the result
result.compute()
Apache Beam provides a unified programming model for both batch and streaming data processing, allowing engineers to implement data pipelines that can run on various execution engines like Apache Flink, Spark, or Google Cloud Dataflow.
These next-generation workflow management systems have improved upon Airflow’s foundation with better developer experience:
- Prefect emphasizes positive engineering with dynamic workflows and built-in failure handling
- Dagster introduces the concept of software-defined assets with rich metadata and dependencies
SQLAlchemy bridges the gap between Python’s object-oriented approach and relational databases, offering both high-level ORM functionality and low-level SQL expression language:
from sqlalchemy import create_engine, Column, Integer, String, Float
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
Base = declarative_base()
class Product(Base):
__tablename__ = 'products'
id = Column(Integer, primary_key=True)
name = Column(String)
price = Column(Float)
# Connect to database
engine = create_engine('postgresql://user:password@localhost/inventory')
Base.metadata.create_all(engine)
# Create session and add data
Session = sessionmaker(bind=engine)
session = Session()
new_product = Product(name='Widget', price=19.99)
session.add(new_product)
session.commit()
Apache Airflow has become the industry standard for orchestrating complex data workflows, allowing engineers to define pipelines as code, monitor execution, and handle dependencies:
from airflow import DAG
from airflow.operators.python import PythonOperator
from datetime import datetime, timedelta
default_args = {
'owner': 'data_engineering',
'depends_on_past': False,
'start_date': datetime(2023, 1, 1),
'email_on_failure': True,
'retries': 3,
'retry_delay': timedelta(minutes=5),
}
dag = DAG(
'daily_sales_processing',
default_args=default_args,
schedule_interval='@daily',
catchup=False
)
def extract_data():
# Code to extract data
pass
def transform_data():
# Code to transform data
pass
def load_data():
# Code to load data
pass
extract_task = PythonOperator(
task_id='extract_sales_data',
python_callable=extract_data,
dag=dag
)
transform_task = PythonOperator(
task_id='transform_sales_data',
python_callable=transform_data,
dag=dag
)
load_task = PythonOperator(
task_id='load_sales_data',
python_callable=load_data,
dag=dag
)
extract_task >> transform_task >> load_task
When selecting programming languages and libraries for your data engineering projects, consider:
- Your team’s existing expertise: Leveraging familiar technologies can accelerate development
- Project requirements: Different tools excel at different scales and use cases
- Integration needs: Ensure compatibility with your existing data ecosystem
- Community support: Larger communities typically mean better documentation and resources
- Performance characteristics: Match tool capabilities to your computational and latency requirements
The most successful data engineering teams typically maintain proficiency in multiple languages and libraries, selecting the right tool for each specific challenge rather than forcing a one-size-fits-all approach.
As the data landscape continues to evolve, staying current with these tools’ capabilities ensures you can build scalable, maintainable data systems that deliver value to your organization.
#DataEngineering #ProgrammingLanguages #Python #Java #Scala #SQL #DataPipelines #Pandas #PySpark #dbt #Airflow #BigData #ETL #DataTransformation #DatabaseManagement #DataScience #DataAnalytics #TechStack #SoftwareDevelopment #DataArchitecture