Jenkins Job Builder: Mastering Configuration as Code for CI/CD Automation
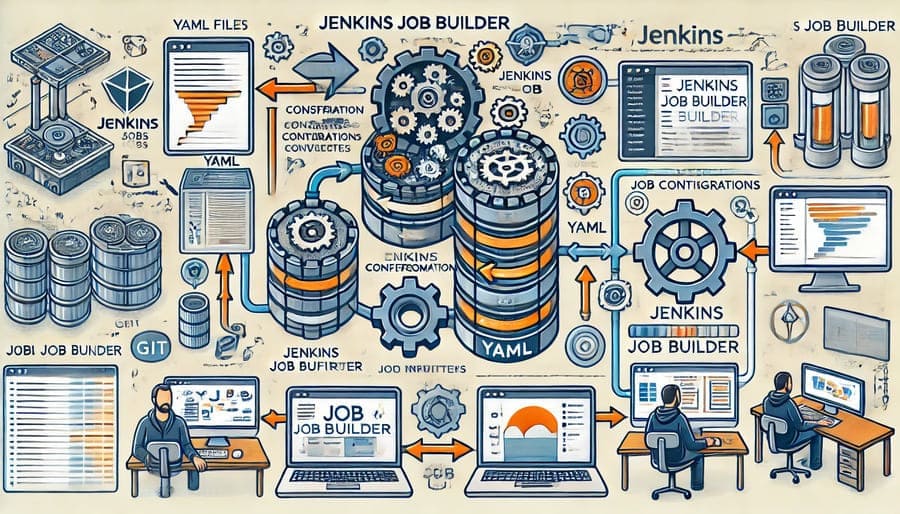
In the rapidly evolving world of DevOps and continuous integration, managing Jenkins jobs efficiently has become a critical challenge for engineering teams. As job configurations grow in number and complexity, maintaining them through the web UI becomes increasingly cumbersome and error-prone. Enter Jenkins Job Builder (JJB)—a powerful system that transforms the way teams define, version, and manage their Jenkins configurations using simple, declarative YAML or JSON files.
Before diving into the specifics of Jenkins Job Builder, it’s important to understand the pain points it addresses. Traditional Jenkins job management often suffers from:
- Configuration Drift: Manual changes made directly in the UI create undocumented differences between environments
- Limited Version Control: UI-based configurations are difficult to track in version control systems
- Repetitive Tasks: Duplicating similar jobs requires tedious copy-pasting and manual adjustments
- Scaling Challenges: As the number of jobs increases, management overhead grows exponentially
- Collaboration Barriers: Team members must have direct Jenkins access to view or modify configurations
Jenkins Job Builder transforms this experience by bringing software engineering best practices to CI/CD configuration management.
Jenkins Job Builder is an open-source Python utility originally developed by the OpenStack community. It allows engineers to define Jenkins jobs as human-readable YAML or JSON files, which are then converted into the XML configuration format that Jenkins uses internally.
This “Configuration as Code” approach provides several key benefits:
- Version Control: Store job definitions alongside application code
- Templating: Create reusable job templates to reduce duplication
- Parameterization: Define variables to generate multiple related jobs
- Review Process: Apply code review workflows to configuration changes
- Testability: Validate configurations before deploying to production
- Documentation: Self-documenting configurations improve knowledge sharing
Setting up Jenkins Job Builder involves a few straightforward steps:
JJB can be installed via pip, the Python package manager:
pip install jenkins-job-builder
Create a configuration file (jenkins_jobs.ini
) to connect JJB to your Jenkins instance:
[jenkins]
url=https://jenkins.example.com
user=admin
password=api_token
For enhanced security, it’s recommended to use Jenkins API tokens instead of passwords.
Create a YAML file defining your first job:
# jobs.yaml
- job:
name: example-job
description: 'A simple example job'
project-type: freestyle
builders:
- shell: |
echo "Hello, Jenkins Job Builder!"
date
echo "Build successful"
Use the JJB command line to update Jenkins with your configuration:
jenkins-jobs --conf jenkins_jobs.ini update jobs.yaml
This command parses your YAML file, converts it to the XML format Jenkins expects, and uploads it to the Jenkins server.
Understanding these fundamental concepts will help you leverage JJB effectively:
Templates allow you to define a job once and reuse it with different parameters:
# Define a template
- job-template:
name: '{project}-{component}-build'
description: 'Build job for {project} {component}'
scm:
- git:
url: 'https://github.com/{organization}/{project}-{component}.git'
branches:
- '{branch}'
builders:
- shell: './build.sh {build-options}'
# Use the template with different parameters
- project:
name: backend-services
organization: example-corp
branch: main
build-options: '--with-tests'
jobs:
- '{project}-{component}-build':
component: api
- '{project}-{component}-build':
component: database
- '{project}-{component}-build':
component: authentication
This example creates three distinct jobs from a single template, demonstrating how JJB can dramatically reduce configuration duplication.
Group related jobs together for better organization:
- job-group:
name: '{name}-jobs'
jobs:
- '{name}-build'
- '{name}-test'
- '{name}-deploy'
- project:
name: user-service
jobs:
- '{name}-jobs'
Define reusable snippets of configuration:
- builder:
name: standard-build-steps
builders:
- shell: |
echo "Running standard build steps"
./gradlew clean build
./run-tests.sh
- job:
name: application-build
builders:
- standard-build-steps
- shell: './generate-docs.sh'
Set default values for common job properties:
- defaults:
name: global
description: 'Job generated by Jenkins Job Builder'
project-type: freestyle
concurrent: true
scm:
- git:
branches:
- main
publishers:
- email:
recipients: '{mail-to}'
- job:
name: example-job
defaults: global
mail-to: team@example.com
builders:
- shell: './build.sh'
Once you’ve mastered the basics, these advanced techniques will help you manage complex Jenkins environments:
JJB fully supports Jenkins Pipeline jobs, both Declarative and Scripted:
- job:
name: deploy-pipeline
project-type: pipeline
dsl: |
pipeline {
agent any
stages {
stage('Build') {
steps {
echo 'Building...'
sh './gradlew build'
}
}
stage('Test') {
steps {
echo 'Testing...'
sh './gradlew test'
}
}
stage('Deploy') {
steps {
echo 'Deploying...'
sh './deploy.sh'
}
}
}
}
For better maintainability, you can also reference external pipeline scripts:
- job:
name: deploy-pipeline
project-type: pipeline
pipeline-scm:
scm:
- git:
url: https://github.com/example/pipelines.git
branches:
- main
script-path: Jenkinsfile
Configure complex multi-branch pipeline jobs:
- job:
name: feature-branch-pipeline
project-type: multibranch
branch-sources:
- git:
remote: 'https://github.com/example/project.git'
credentials-id: 'github-credentials'
orphaned-item-strategy:
days-to-keep: 7
factory:
workflow-branch-project:
script-path: Jenkinsfile
Organize jobs into views for better navigation:
- view:
name: Frontend Projects
view-type: list
job-name:
- 'frontend-.*'
columns:
- status
- weather
- job
- last-success
- last-failure
- last-duration
Create test matrices to run jobs across multiple dimensions:
- job:
name: cross-platform-tests
project-type: matrix
axes:
- axis:
type: slave
name: os
values:
- linux
- windows
- macos
- axis:
type: user-defined
name: browser
values:
- chrome
- firefox
- safari
builders:
- shell: './run-tests.sh ${os} ${browser}'
Structure jobs in folders for cleaner organization:
- job:
name: services/authentication/build
project-type: freestyle
builders:
- shell: './build.sh'
- job:
name: services/authentication/deploy
project-type: freestyle
builders:
- shell: './deploy.sh'
Jenkins Job Builder integrates seamlessly with modern DevOps practices:
Store job definitions alongside application code or in a dedicated infrastructure repository:
repo-structure/
├── src/
├── tests/
├── jenkins/
│ ├── jobs/
│ │ ├── build.yaml
│ │ ├── test.yaml
│ │ └── deploy.yaml
│ ├── templates/
│ │ └── common.yaml
│ └── jenkins_jobs.ini
└── README.md
Implement continuous deployment of Jenkins configurations:
# In your CI pipeline configuration
stages:
- test
- deploy
test-jjb-configs:
stage: test
script:
- jenkins-jobs test jenkins/jobs/*.yaml
deploy-jjb-configs:
stage: deploy
script:
- jenkins-jobs --conf jenkins/jenkins_jobs.ini update jenkins/jobs/*.yaml
only:
- main
Generate job configurations dynamically based on repository structure or metadata:
import yaml
import os
# Scan repositories for services
services = [d for d in os.listdir('./services') if os.path.isdir(f'./services/{d}')]
jobs = []
for service in services:
# Create a job definition for each service
job = {
'job': {
'name': f'{service}-build',
'project-type': 'freestyle',
'scm': [{
'git': {
'url': f'https://github.com/example/{service}.git',
'branches': ['main']
}
}],
'builders': [{
'shell': './build.sh'
}]
}
}
jobs.append(job)
# Write the generated configurations to a YAML file
with open('generated-jobs.yaml', 'w') as f:
yaml.dump_all(jobs, f, default_flow_style=False)
Adopt these patterns to make your JJB configurations maintainable and scalable:
- Use Hierarchical Naming: Adopt a consistent naming convention, such as
{service}-{component}-{action}
- Group Related Files: Organize jobs by project, team, or function
- Separate Templates and Jobs: Keep reusable templates in dedicated files
- DRY (Don’t Repeat Yourself): Use templates, macros, and defaults extensively
- Parameterize Everything: Make configurations flexible with variables
- Keep it Simple: Avoid complex nested structures when possible
- Document Inline: Add comments to explain non-obvious configurations
- Review Changes: Implement pull requests for configuration changes
- Test Before Deployment: Use the
test
command to validate configurations - Incremental Updates: Update specific jobs rather than all jobs when possible
- Track History: Maintain a changelog for significant configuration changes
- Credential Management: Avoid hardcoding secrets in job definitions
- Use Jenkins Credential IDs: Reference credential IDs rather than including actual credentials
- Implement Least Privilege: Ensure the JJB user has only the permissions it needs
Even with a well-designed system, issues can arise. Here’s how to address common problems:
If JJB reports validation errors:
- Check YAML syntax for proper indentation and structure
- Verify that all required fields are present
- Ensure referenced templates and macros are defined
When jobs fail to update:
- Verify Jenkins connection details in the configuration file
- Check that the Jenkins user has sufficient permissions
- Review Jenkins logs for specific error messages
- Use
--debug
flag for verbose output:jenkins-jobs --debug update jobs.yaml
For problems with template variables:
- Confirm that all variables used in templates are defined
- Check for typos in variable names
- Verify the correct syntax for variable references (
{variable-name}
)
If the generated XML is incorrect:
- Use the
test
command to inspect the output:jenkins-jobs test jobs.yaml
- Compare with XML exported directly from Jenkins
- Check JJB documentation for the specific plugin or feature you’re configuring
As the DevOps landscape evolves, Jenkins Job Builder continues to adapt:
- Expanded Plugin Support: Coverage for newer Jenkins plugins
- Cloud Integration: Enhanced support for cloud-based Jenkins deployments
- Pipeline Enhancements: Better tooling for complex pipeline jobs
- Infrastructure as Code Convergence: Tighter integration with tools like Terraform and Ansible
- UI Improvements: Development of better visualization and management tools
Jenkins Job Builder represents a paradigm shift in how teams manage their CI/CD infrastructure. By bringing software engineering principles to Jenkins configuration, it addresses the scalability and maintainability challenges that have long plagued Jenkins administrators.
For engineering organizations looking to adopt DevOps best practices, JJB offers a path to treat “pipeline as code” with the same rigor as application code. The result is more reliable, repeatable, and collaborative CI/CD processes that can evolve alongside your applications.
Whether you’re managing a handful of jobs or hundreds, Jenkins Job Builder provides the tools to bring order to complexity, enabling your team to focus on building and delivering software rather than maintaining CI/CD configurations.
Keywords: Jenkins Job Builder, configuration as code, YAML Jenkins configuration, Jenkins automation, CI/CD infrastructure, Jenkins jobs management, DevOps tools, Jenkins templates, pipeline as code, infrastructure automation
Hashtags: #JenkinsJobBuilder #ConfigurationAsCode #DevOps #CI/CD #JenkinsAutomation #InfrastructureAsCode #PipelineAutomation #YAML #JenkinsConfig #DevOpsTools