Temporal: Revolutionizing Microservice Orchestration for Reliable Distributed Systems
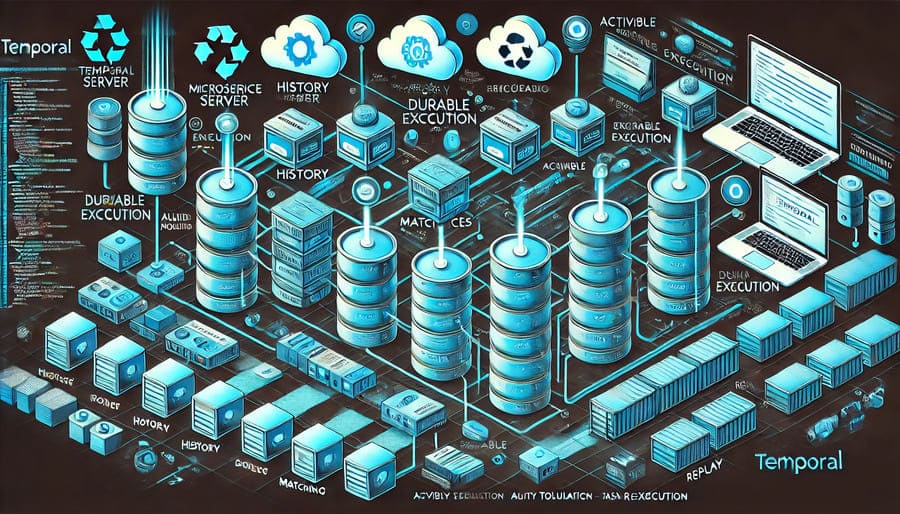
In today’s increasingly complex world of distributed systems, building reliable, scalable applications has become one of the most significant challenges facing developers and architects. Enter Temporal—an open-source microservice orchestration platform designed to solve the hardest technical problems in distributed systems while radically simplifying developer experience. Born from the lessons learned at Uber with its predecessor Cadence, Temporal has rapidly gained adoption across industries for its unique approach to handling reliability, scalability, and complexity in distributed systems.
Before diving into Temporal’s capabilities, it’s essential to understand the problems it addresses. Modern applications built on microservice architectures face several critical challenges:
- Reliability: Services fail, networks are unreliable, and systems crash. How do you ensure workflows complete despite these failures?
- State Management: Tracking the state of long-running processes across distributed components is notoriously difficult.
- Complex Coordination: Orchestrating interactions between multiple services while handling retries, timeouts, and backoffs.
- Visibility: Understanding what’s happening in distributed workflows is often a blind spot.
- Development Complexity: Building reliable distributed systems traditionally requires complex distributed systems expertise.
These challenges have historically required extensive engineering resources and specialized knowledge to address effectively. Temporal’s innovation lies in providing a comprehensive solution to these problems while maintaining a developer-friendly experience.
Temporal is a microservice orchestration platform that provides a programming model, runtime, and service for building reliable applications. At its core, Temporal enables developers to write code that is guaranteed to complete execution despite failures in the underlying infrastructure.
Temporal introduces several key concepts that form the foundation of its approach:
In Temporal, workflows are durable, reliable functions that contain the business logic of your application. Unlike regular functions, Temporal workflows can run for seconds, days, or even years, surviving process, node, and even data center failures. Workflows are defined in code as ordinary functions but gain extraordinary powers through the Temporal SDK.
// Example workflow in Go
func OrderFulfillmentWorkflow(ctx workflow.Context, orderID string) error {
logger := workflow.GetLogger(ctx)
logger.Info("Order fulfillment workflow started", "orderID", orderID)
// Payment processing
var paymentResult PaymentResult
paymentCtx := workflow.WithActivityOptions(ctx, workflow.ActivityOptions{
ScheduleToStartTimeout: time.Minute,
StartToCloseTimeout: time.Minute * 5,
RetryPolicy: &temporal.RetryPolicy{
InitialInterval: time.Second,
BackoffCoefficient: 2.0,
MaximumInterval: time.Minute * 5,
MaximumAttempts: 10,
},
})
err := workflow.ExecuteActivity(paymentCtx, ProcessPaymentActivity, orderID).Get(paymentCtx, &paymentResult)
if err != nil {
return err
}
// Inventory reservation
var inventoryResult InventoryResult
err = workflow.ExecuteActivity(paymentCtx, ReserveInventoryActivity, orderID, paymentResult.Items).Get(paymentCtx, &inventoryResult)
if err != nil {
// If inventory fails, we need to refund the payment
compensationCtx := workflow.WithActivityOptions(ctx, workflow.ActivityOptions{
StartToCloseTimeout: time.Minute * 5,
})
workflow.ExecuteActivity(compensationCtx, RefundPaymentActivity, orderID, paymentResult.TransactionID)
return err
}
// Shipping and notification can happen in parallel
shippingCtx := workflow.WithActivityOptions(ctx, workflow.ActivityOptions{
StartToCloseTimeout: time.Hour * 24,
})
// Future representing the shipping process
shippingFuture := workflow.ExecuteActivity(shippingCtx, ArrangeShippingActivity, orderID, inventoryResult.Items)
// Process notifications while shipping is being arranged
notificationCtx := workflow.WithActivityOptions(ctx, workflow.ActivityOptions{
StartToCloseTimeout: time.Minute * 5,
})
err = workflow.ExecuteActivity(notificationCtx, SendOrderConfirmationActivity, orderID).Get(notificationCtx, nil)
if err != nil {
logger.Warn("Failed to send notification, but continuing workflow", "error", err)
}
// Now wait for shipping to complete
var shippingResult ShippingResult
err = shippingFuture.Get(shippingCtx, &shippingResult)
if err != nil {
return err
}
logger.Info("Order fulfillment completed successfully", "orderID", orderID)
return nil
}
Activities are the basic unit of work in Temporal. They represent individual steps within a workflow and are executed as separate processes. Activities can call external APIs, access databases, or interact with other services. Unlike workflows, activities do not need to be deterministic, making them suitable for side effects and integrations.
// Example activity in Go
func ProcessPaymentActivity(ctx context.Context, orderID string) (PaymentResult, error) {
logger := activity.GetLogger(ctx)
logger.Info("Processing payment", "orderID", orderID)
// Fetch order details from database
order, err := orderService.GetOrder(orderID)
if err != nil {
return PaymentResult{}, err
}
// Call payment provider API
paymentResult, err := paymentProvider.ProcessPayment(order.CustomerID, order.Amount, order.PaymentMethod)
if err != nil {
logger.Error("Payment processing failed", "error", err)
return PaymentResult{}, err
}
// Record transaction in database
err = orderService.UpdatePaymentStatus(orderID, paymentResult.TransactionID, "completed")
if err != nil {
// We need to handle this carefully - payment was processed but we failed to record it
logger.Error("Failed to update payment status in database", "error", err)
return PaymentResult{}, err
}
return PaymentResult{
TransactionID: paymentResult.TransactionID,
Status: "completed",
Items: order.Items,
}, nil
}
Workers are long-running processes that execute workflow and activity code. They pull tasks from the Temporal service, execute them, and return the results. Workers can be scaled horizontally to handle increased load, and they can be implemented in any language supported by the Temporal SDK.
// Setting up a worker in Go
func main() {
c, err := client.NewClient(client.Options{})
if err != nil {
log.Fatalln("Unable to create client", err)
}
defer c.Close()
w := worker.New(c, "fulfillment-tasks", worker.Options{})
// Register workflow and activities
w.RegisterWorkflow(OrderFulfillmentWorkflow)
w.RegisterActivity(ProcessPaymentActivity)
w.RegisterActivity(ReserveInventoryActivity)
w.RegisterActivity(RefundPaymentActivity)
w.RegisterActivity(ArrangeShippingActivity)
w.RegisterActivity(SendOrderConfirmationActivity)
// Start the worker
err = w.Run(worker.InterruptCh())
if err != nil {
log.Fatalln("Unable to start worker", err)
}
}
The Temporal Service is the central component that coordinates workflow execution. It maintains workflow state, dispatches tasks to workers, handles failures, and provides visibility into workflow execution. The service consists of several core components:
- Frontend Gateway: Handles API requests from clients and workers
- History Service: Maintains workflow execution history
- Matching Service: Routes tasks to appropriate workers
- Worker Service: Manages workflow timers and events
- Persistence Layer: Stores workflow history and state
Temporal’s most powerful feature is durable execution. When a workflow executes, every state transition is recorded in the workflow history. If a worker fails during execution, another worker can pick up the workflow and continue from where it left off. This means that your business logic is guaranteed to complete execution, regardless of failures in the underlying infrastructure.
As applications evolve, workflow definitions change. Temporal provides built-in versioning mechanisms that allow you to update workflow code while ensuring that in-flight executions complete successfully using the code version they started with.
// Example of workflow versioning in Go
func WorkflowWithVersioning(ctx workflow.Context, input string) (string, error) {
logger := workflow.GetLogger(ctx)
// Check workflow version
v := workflow.GetVersion(ctx, "FeatureFlag", workflow.DefaultVersion, 1)
if v == workflow.DefaultVersion {
// Original implementation
logger.Info("Running original implementation")
result, err := workflow.ExecuteActivity(ctx, OriginalActivity, input).Get(ctx, nil)
if err != nil {
return "", err
}
return result.(string), nil
} else {
// New implementation
logger.Info("Running new implementation")
result, err := workflow.ExecuteActivity(ctx, ImprovedActivity, input).Get(ctx, nil)
if err != nil {
return "", err
}
return result.(string) + " (improved)", nil
}
}
Temporal provides detailed visibility into workflow execution through its Web UI and API. You can see the current state of a workflow, its execution history, and any errors that occurred during execution. This visibility is invaluable for debugging and monitoring complex distributed applications.
Temporal supports multiple programming languages through its SDKs, including:
- Go
- Java
- TypeScript/JavaScript
- PHP
- Python (community-maintained)
- .NET (community-maintained)
This language flexibility allows teams to implement workflows in the language that best suits their expertise and existing infrastructure.
Temporal makes it easy to implement the Saga pattern for managing distributed transactions. The platform’s guaranteed execution model ensures that compensating actions are properly executed in case of failures.
// Saga pattern example in TypeScript
async function bookingWorkflow(ctx: Workflow, input: BookingInput): Promise<BookingResult> {
const saga = new Saga({
ctx,
continueWithError: false,
});
try {
// Book flight
const flightReservation = await executeActivity('bookFlight', {
scheduleToCloseTimeout: '1h',
}, {
flightDetails: input.flightDetails,
userId: input.userId,
});
// Add compensation in case we need to roll back
saga.addCompensation('cancelFlight', {
reservationId: flightReservation.id,
});
// Book hotel
const hotelReservation = await executeActivity('bookHotel', {
scheduleToCloseTimeout: '1h',
}, {
hotelDetails: input.hotelDetails,
userId: input.userId,
dates: input.dates,
});
// Add compensation
saga.addCompensation('cancelHotel', {
reservationId: hotelReservation.id,
});
// Book car
const carReservation = await executeActivity('bookCar', {
scheduleToCloseTimeout: '1h',
}, {
carDetails: input.carDetails,
userId: input.userId,
dates: input.dates,
});
// Add compensation
saga.addCompensation('cancelCar', {
reservationId: carReservation.id,
});
// Process payment
await executeActivity('processPayment', {
scheduleToCloseTimeout: '10m',
}, {
userId: input.userId,
amount: flightReservation.cost + hotelReservation.cost + carReservation.cost,
});
return {
bookingId: generateUUID(),
status: 'CONFIRMED',
flightReservationId: flightReservation.id,
hotelReservationId: hotelReservation.id,
carReservationId: carReservation.id,
};
} catch (error) {
// If any step fails, execute compensations in reverse order
await saga.compensate();
throw error;
}
}
Temporal’s flexibility and reliability make it suitable for a wide range of applications across various industries:
E-commerce platforms use Temporal to orchestrate the entire order fulfillment process, from payment processing to inventory management, shipping, and notifications. The platform’s durability ensures that orders are never lost, even in the face of system failures.
Financial institutions leverage Temporal for transaction processing, fraud detection, and compliance workflows. The platform’s strong consistency guarantees and ability to maintain state over long periods make it ideal for financial applications where reliability is paramount.
In healthcare, Temporal orchestrates patient care workflows, insurance verification, and medical record processing. The ability to handle long-running processes while maintaining compliance with regulations makes Temporal a natural fit for healthcare applications.
DevOps teams use Temporal to build reliable CI/CD pipelines, infrastructure provisioning workflows, and deployment processes. The platform’s error handling and retry capabilities ensure that infrastructure changes are applied consistently.
Supply chain applications leverage Temporal to coordinate complex logistics processes, inventory management, and vendor interactions. The ability to maintain state across multiple systems and handle long-running processes makes Temporal well-suited for supply chain orchestration.
While both Temporal and Airflow are workflow orchestration platforms, they serve different purposes:
- Programming Model: Temporal uses code-first workflows, while Airflow uses DAG configurations
- Execution Model: Temporal provides durable execution guarantees, while Airflow focuses on scheduled task execution
- Use Case Focus: Temporal excels at distributed microservice orchestration, while Airflow specializes in data pipeline orchestration
- State Management: Temporal has built-in state management, while Airflow requires external storage for state
Some teams build orchestration solutions using Kafka and stateful services:
- Development Complexity: Temporal provides a higher-level abstraction, reducing development complexity
- Durability Guarantees: Temporal handles durability out of the box, while Kafka-based solutions require custom implementations
- Visibility: Temporal offers superior visibility into workflow execution
- Feature Set: Temporal includes built-in workflow versioning, retries, and timeouts
Many organizations have built custom state machine-based workflow engines:
- Maintenance Burden: Temporal reduces the maintenance burden of custom solutions
- Feature Richness: Temporal provides a more comprehensive feature set out of the box
- Community Support: Temporal has a growing community and ecosystem
- Scalability: Temporal’s architecture is designed for horizontal scalability
Setting up a basic Temporal environment involves a few key steps:
The easiest way to get started is using the Docker Compose setup:
git clone https://github.com/temporalio/docker-compose.git
cd docker-compose
docker-compose up
This starts a local Temporal service with all required dependencies.
Here’s a simple example of a Temporal application in TypeScript:
// workflow.ts
import { proxyActivities, defineWorkflow } from '@temporalio/workflow';
// Define activities
const activities = proxyActivities({
startToCloseTimeout: '1 minute',
});
// Define workflow
export const greetingWorkflow = defineWorkflow(async (name: string): Promise<string> => {
const greeting = await activities.generateGreeting(name);
return greeting;
});
// activities.ts
export async function generateGreeting(name: string): Promise<string> {
return `Hello, ${name}!`;
}
// worker.ts
import { Worker } from '@temporalio/worker';
import * as activities from './activities';
import { greetingWorkflow } from './workflow';
async function run() {
const worker = await Worker.create({
workflowsPath: require.resolve('./workflow'),
activities,
taskQueue: 'greeting-tasks',
});
await worker.run();
}
run().catch((err) => {
console.error(err);
process.exit(1);
});
// client.ts
import { Connection, Client } from '@temporalio/client';
import { greetingWorkflow } from './workflow';
async function run() {
const connection = await Connection.connect();
const client = new Client({ connection });
// Start workflow execution
const handle = await client.workflow.start(greetingWorkflow, {
args: ['Temporal'],
taskQueue: 'greeting-tasks',
workflowId: 'greeting-workflow-' + Date.now(),
});
console.log(`Started workflow ${handle.workflowId}`);
// Wait for workflow to complete
const result = await handle.result();
console.log(result); // Hello, Temporal!
}
run().catch((err) => {
console.error(err);
process.exit(1);
});
Start the worker and client in separate terminals:
# Terminal 1
npm run worker
# Terminal 2
npm run client
- Keep Workflows Deterministic: Avoid non-deterministic operations like random number generation or time-based logic in workflows
- Limit Workflow Logic: Focus workflow code on orchestration, delegating business logic to activities
- Define Appropriate Timeouts: Set realistic timeouts for activities based on their expected execution time
- Use Signals for External Events: Leverage signals to handle external events that should affect workflow execution
- Implement Idempotent Activities: Design activities to be idempotent to handle retries safely
- Scale Workers Appropriately: Monitor worker metrics and scale based on task queue depth
- Set Up Proper Monitoring: Implement alerts for workflow failures and SLA violations
- Use Namespaces for Isolation: Separate different applications or environments using Temporal namespaces
- Implement Graceful Worker Shutdown: Handle worker shutdowns properly to avoid workflow interruption
- Plan for Disaster Recovery: Configure appropriate backup and recovery procedures for the Temporal service
- Test Workflows Thoroughly: Use Temporal’s testing framework to verify workflow behavior
- Implement Versioning Strategy: Plan for workflow code changes using Temporal’s versioning features
- Use Workflow ID Reuse Policy: Configure appropriate reuse policies based on your application’s needs
- Leverage Child Workflows: Break down complex processes into parent and child workflows
- Document Workflow Behavior: Maintain clear documentation of workflow purpose and behavior
As distributed systems continue to evolve, Temporal is positioned to address emerging challenges:
Temporal is developing tighter integration with serverless platforms, allowing workflows to orchestrate functions across different providers while maintaining durability guarantees.
Future versions will provide even more comprehensive visibility into workflow execution, with improved debugging tools, anomaly detection, and performance analysis capabilities.
Temporal is enhancing its support for multi-region deployments, enabling global workflow execution with cross-region reliability and data sovereignty compliance.
Improved support for data schema evolution will make it easier to handle changes in workflow input and output data structures over time.
The Temporal community continues to develop and maintain SDKs for additional programming languages, expanding the platform’s accessibility to more development teams.
Temporal represents a significant advancement in microservice orchestration, addressing the fundamental challenges of building reliable distributed systems. By providing a programming model that abstracts away the complexities of distributed state management, retries, and error handling, Temporal allows developers to focus on business logic while ensuring their applications remain resilient in the face of failures.
For organizations building microservice architectures, Temporal offers a compelling solution that reduces development time, improves reliability, and provides unprecedented visibility into distributed processes. As distributed systems become increasingly prevalent, platforms like Temporal that simplify their development and operation will become essential tools in the modern software engineering toolkit.
Whether you’re building e-commerce systems, financial services, healthcare applications, or DevOps workflows, Temporal’s approach to reliable execution and state management can help you build more robust, maintainable, and scalable distributed applications.
Keywords: Temporal platform, microservice orchestration, distributed systems, workflow automation, durable execution, saga pattern, reliable services, microservice coordination, fault-tolerant workflows, distributed transactions
Hashtags: #Temporal #MicroserviceOrchestration #DistributedSystems #WorkflowAutomation #FaultTolerance #ReliableServices #Orchestration #Microservices #DurableExecution #SagaPattern