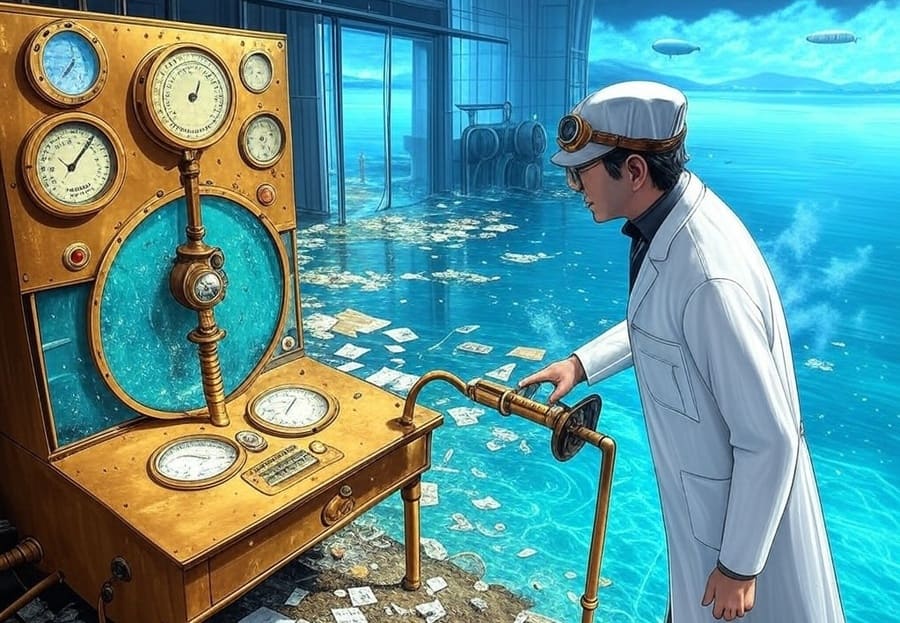
In the modern data landscape, organizations are drowning in data while thirsting for insights. Traditional data lakes promised a solution but delivered a new set of challenges: data quality issues, reliability problems, and performance bottlenecks that left many teams frustrated and unable to extract true value from their data investments.
Enter Delta Lake – an open-source storage layer that brings reliability and performance to data lakes. After implementing Delta Lake across multiple enterprise environments, I’ve seen firsthand how it transforms chaotic data lakes into reliable, high-performance data platforms. In this guide, I’ll walk through why Delta Lake matters and how to implement it effectively, even if you’re just getting started.
Before diving into Delta Lake, let’s understand why traditional data lakes often become “data swamps”:
- No ACID Transactions: Traditional data lakes lack atomicity, consistency, isolation, and durability guarantees.
- Schema Enforcement Challenges: Without schema validation, corrupt or invalid data easily pollutes your lake.
- Limited Support for Streaming: Batch and streaming workflows often require separate architectures.
- Inefficient Processing: Performance degrades as data volumes grow due to small files and limited metadata.
- Complex Time Travel & Audit: Reproducing historical states or auditing changes becomes nearly impossible.
I once worked with a financial services company that had invested heavily in a traditional data lake architecture, only to discover they couldn’t trust their data for regulatory reporting because they couldn’t guarantee its consistency or track changes over time. That’s a costly problem that Delta Lake directly solves.
Delta Lake addresses these challenges through several key capabilities:
Delta Lake provides ACID guarantees through a transaction log that tracks all changes to your data. This log is the heart of Delta Lake – every operation is recorded as a transaction, ensuring data consistency even if a job fails midway through execution.
Here’s what the Delta transaction log looks like under the hood:
_delta_log/
00000000000000000000.json
00000000000000000001.json
00000000000000000002.json
...
Each JSON file represents an atomic, consistent transaction. If we examined a transaction file, we might see:
{"commitInfo":{"timestamp":1664834025142,"operation":"WRITE",...}}
{"add":{"path":"part-00000-af0c3312-13a2-4f79-8fb2-9e625be7948e-c000.snappy.parquet","size":97457,"partitionValues":{"date":"2023-01-01"},...}}
This detailed tracking enables Delta Lake to provide consistency guarantees that traditional data lakes simply cannot match.
Delta Lake validates that incoming data matches your table’s schema, preventing corrupt data from polluting your lake. When your data requirements change, you can explicitly evolve your schema with commands like:
# Add a new column with a default value
spark.sql("ALTER TABLE customer_data ADD COLUMN (loyalty_tier STRING DEFAULT 'Bronze')")
# Merge schema changes automatically when appropriate
df.write.option("mergeSchema", "true").format("delta").mode("append").save("/data/customer_data")
This combination of enforcement and flexibility means your data remains reliable over time, even as business requirements evolve.
One of Delta Lake’s most powerful features is the ability to access previous versions of your data – something that’s nearly impossible with traditional data lakes. This capability is invaluable for audit, compliance, and debugging purposes.
# Query the table as it was 5 versions ago
df = spark.read.format("delta").option("versionAsOf", 5).load("/data/customer_data")
# Query the table as it was at a specific timestamp
df = spark.read.format("delta").option("timestampAsOf", "2023-01-01T00:00:00.000Z").load("/data/customer_data")
This has saved countless hours in my projects – from recovering from erroneous data updates to responding to regulatory audit requests that required historical data states.
Delta Lake includes several optimizations that dramatically improve query performance:
- Data Skipping: Delta Lake maintains statistics about each data file, allowing it to skip files that don’t match query predicates.
- Z-Ordering: Multi-dimensional clustering that co-locates related data.
- Compaction: Combines small files to avoid performance degradation from file fragmentation.
These optimizations often yield 10-100x performance improvements for analytical queries compared to traditional data lakes.
Let’s walk through how to implement Delta Lake in your environment, with practical examples.
Delta Lake works with Apache Spark, so you’ll need a Spark environment. You can use Databricks (which includes Delta Lake natively), or add Delta Lake to your own Spark environment:
For Maven/SBT:
<dependency>
<groupId>io.delta</groupId>
<artifactId>delta-core_2.12</artifactId>
<version>2.3.0</version>
</dependency>
For PySpark:
pip install delta-spark
Initialize Spark with Delta Lake:
from pyspark.sql import SparkSession
spark = SparkSession.builder \
.appName("DeltaLakeDemo") \
.config("spark.sql.extensions", "io.delta.sql.DeltaSparkSessionExtension") \
.config("spark.sql.catalog.spark_catalog", "org.apache.spark.sql.delta.catalog.DeltaCatalog") \
.getOrCreate()
Converting existing data to Delta format is straightforward:
# From a DataFrame
df = spark.read.csv("/data/raw/sales.csv", header=True, inferSchema=True)
df.write.format("delta").save("/data/delta/sales")
# From an existing Parquet table
spark.sql("CONVERT TO DELTA parquet.`/data/parquet/customers`")
# Create a Delta table directly from SQL
spark.sql("""
CREATE TABLE IF NOT EXISTS sales_delta
USING DELTA
LOCATION '/data/delta/sales'
AS SELECT * FROM sales_parquet
""")
Now let’s leverage Delta Lake’s ACID capabilities with practical operations:
# Append new sales data
new_sales.write.format("delta").mode("append").save("/data/delta/sales")
# Insert using SQL
spark.sql("""
INSERT INTO sales_delta
VALUES (1001, 'Product X', 299.99, '2023-01-15')
""")
from delta.tables import DeltaTable
# Update using Python API
delta_table = DeltaTable.forPath(spark, "/data/delta/sales")
delta_table.update(
condition = "product_id = '1001'",
set = {"price": "399.99"}
)
# Update using SQL
spark.sql("""
UPDATE sales_delta
SET price = 399.99
WHERE product_id = '1001'
""")
# Delete using Python API
delta_table.delete("product_id = '1001'")
# Delete using SQL
spark.sql("DELETE FROM sales_delta WHERE product_id = '1001'")
This is particularly powerful for handling data that requires both updates and inserts:
from pyspark.sql.functions import col
# Merge operation
delta_table.alias("target").merge(
source = updates_df.alias("source"),
condition = "target.product_id = source.product_id"
).whenMatchedUpdate(
set = {"price": "source.price", "updated_date": "current_timestamp()"}
).whenNotMatchedInsert(
values = {
"product_id": "source.product_id",
"product_name": "source.product_name",
"price": "source.price",
"created_date": "current_timestamp()"
}
).execute()
Delta Lake provides several commands to maintain performance as your data grows:
# Compact small files
spark.sql("OPTIMIZE sales_delta")
# Z-Order by frequently filtered columns
spark.sql("OPTIMIZE sales_delta ZORDER BY (date, product_id)")
# Vacuum old files (remove files not needed by current version)
# Default retention is 7 days
spark.sql("VACUUM sales_delta RETAIN 168 HOURS")
As your data evolves, Delta Lake can adapt:
# Add a new column
spark.sql("ALTER TABLE sales_delta ADD COLUMN promotion_id STRING")
# Enable schema merging when writing with a new schema
df_with_new_column.write \
.format("delta") \
.option("mergeSchema", "true") \
.mode("append") \
.save("/data/delta/sales")
Delta Lake’s time travel capabilities are invaluable for auditing, debugging, and compliance:
# View table history
spark.sql("DESCRIBE HISTORY sales_delta").show()
# Query previous version
df_v5 = spark.read.format("delta").option("versionAsOf", 5).load("/data/delta/sales")
# Restore to previous version
delta_table.restore(version=5)
Let me share a real implementation I worked on (with names changed for privacy).
A retail analytics company was struggling with their data lake – inconsistent data, slow queries, and an inability to track historical changes were undermining their analytics products.
We implemented Delta Lake with a focus on their critical customer behavior tables, which received both batch and streaming updates. Here’s what the implementation looked like:
- Ingestion Layer:
- Converted raw Kafka streams to Delta format using Structured Streaming
- Implemented schema validation to catch data issues early
- Silver Layer:
- Created cleansed tables with business rules applied
- Used Delta’s merge operations to handle late-arriving data
- Implemented quality checks that rolled back transactions if data failed validation
- Gold Layer:
- Built aggregated analytics tables optimized for query performance
- Used Z-Ordering on frequently filtered dimensions
- Implemented automatic compaction jobs to maintain performance
The results were dramatic:
- Query performance improved by 60% on average
- Data reliability issues decreased by 90%
- Development time for new features dropped by 40% as engineers spent less time troubleshooting data issues
- Compliance requirements for historical data access were satisfied using time travel
The most impactful aspect? Their data scientists could finally trust the data they were building models on, resulting in more accurate predictions and better business outcomes.
Based on my experience implementing Delta Lake across different organizations, here are key pitfalls to avoid:
Problem: Too many small files kill performance over time. Solution: Schedule regular OPTIMIZE commands to compact files and maintain an average file size of 1GB.
# Schedule this to run daily on large tables
spark.sql("OPTIMIZE sales_delta WHERE date_trunc('day', timestamp) = current_date() - interval 1 day")
Problem: Z-Ordering is powerful but computationally expensive. Solution: Only Z-Order on columns frequently used in filters and limit to 3-4 columns maximum.
Problem: Old file versions accumulate, consuming storage and degrading performance. Solution: Implement regular VACUUM operations with appropriate retention policies.
# Caution: Ensure retention period meets your time travel needs
spark.sql("VACUUM sales_delta RETAIN 30 DAYS")
Problem: Poor partition choices lead to performance issues. Solution: Partition on columns that distribute data evenly and are commonly used in filters.
# Good partitioning for time-series data
df.write.format("delta").partitionBy("year", "month").save("/data/delta/sales")
Delta Lake excels in many scenarios, but it’s not always the right choice:
Ideal for Delta Lake:
- Data lakes with multiple readers and writers
- Environments requiring ACID transactions
- Use cases needing historical data access
- Mixed batch and streaming workloads
- Scenarios requiring schema enforcement
- Analytical workloads with varying query patterns
May not be ideal for:
- Simple, read-only data stores with limited concurrency
- Very high-throughput, low-latency transaction processing (OLTP)
- Extremely small datasets where overhead isn’t justified
- Environments where Apache Spark isn’t available
If you’re ready to implement Delta Lake, here’s a practical roadmap:
- Start small – convert a single important table to Delta format
- Implement basic operations and observe the improvements
- Gradually expand to related tables, prioritizing those with reliability or performance issues
- Develop best practices for your organization around partitioning, optimization, and retention
- Train your team on Delta Lake concepts and maintenance
Delta Lake isn’t just a technical improvement – it represents a paradigm shift toward the “lakehouse” architecture, combining the best elements of data warehouses (reliability, performance, ACID transactions) with the flexibility and scalability of data lakes.
By implementing Delta Lake, you’re not just solving today’s data problems; you’re building the foundation for a modern data architecture that can adapt to tomorrow’s challenges.
The question isn’t whether you can afford to implement Delta Lake – it’s whether you can afford not to. In a world where data is the new oil, Delta Lake is the refinery that transforms raw potential into actual business value.
What challenges are you facing with your data lake implementation? Share in the comments, and I’ll provide specific guidance on how Delta Lake might help address them.
DeltaLake #DataLakehouse #ApacheSpark #DataEngineering #ACID #DataReliability #BigData #DataArchitecture #Databricks #OpenSource #DataLake #ETL #DataOps #CloudData #SchemaEvolution #TimeTravel #DataGovernance #SparkSQL #DataProcessing #AnalyticsEngineering